用python写一个ros2里小乌龟画圆的代码
时间: 2024-05-04 10:21:41 浏览: 222
好的,以下是用Python编写在ROS2中让小乌龟画圆的代码:
```
# 导入需要的 Python 第三方库和 ROS2 模块
import math
import rclpy
from geometry_msgs.msg import Twist
from turtlesim.msg import Pose
# 初始化小乌龟的运动节点
class TurtleMotion:
def __init__(self):
# 创建节点对象
self.node = rclpy.create_node('turtle_motion')
# 订阅小乌龟的位置
self.sub = self.node.create_subscription(Pose,'/turtle1/pose',self.pose_callback,10)
# 发布小乌龟的速度
self.pub = self.node.create_publisher(Twist,'/turtle1/cmd_vel',10)
# 设置小乌龟的线速度和角速度
self.tw = Twist()
self.tw.linear.x = 0.5
self.tw.angular.z = 0.5 * math.pi / 180.0
# 定义小乌龟的方向和运动状态
self.current_direction = 0
self.move_state = 'forward'
# 开始小乌龟的运动
self.motion()
# 小乌龟的位置回调函数
def pose_callback(self, data):
# 判断小乌龟是否走出边界
if (data.x > 11.0 or data.x < 0.1 or data.y > 11.0 or data.y < 0.1):
self.node.get_logger().warn('out of boundary')
self.node.destroy_node()
rclpy.shutdown()
else:
# 判断小乌龟当前方向与运动状态
if (self.current_direction == 0 and self.move_state == 'forward'):
if (data.theta >= 0 and data.theta < math.pi / 2.0):
self.current_direction = 1
elif (self.current_direction == 1 and self.move_state == 'forward'):
if (data.theta >= math.pi / 2.0 and data.theta < math.pi):
self.current_direction = 2
elif (self.current_direction == 2 and self.move_state == 'forward'):
if (data.theta >= -math.pi and data.theta < -math.pi / 2.0):
self.current_direction = 3
elif (self.current_direction == 3 and self.move_state == 'forward'):
if (data.theta >= -math.pi / 2.0 and data.theta < 0):
self.current_direction = 0
self.move_state = 'finish'
# 小乌龟的运动函数
def motion(self):
# 发布速度消息,让小乌龟开始运动
self.pub.publish(self.tw)
# 循环检查小乌龟运动状态
while rclpy.ok():
# 如果小乌龟到达圆心位置,则停止运动
if (self.move_state == 'finish'):
self.tw.linear.x = 0
self.tw.angular.z = 0
self.pub.publish(self.tw)
break
# 运行小乌龟运动节点
def main(args=None):
rclpy.init(args=args)
turtle_motion = TurtleMotion()
rclpy.spin(turtle_motion.node)
turtle_motion.node.destroy_node()
rclpy.shutdown()
if __name__ == '__main__':
main()
```
这段代码的作用是定义了一个节点 `turtle_motion`,并通过 ROS2 中的 Twist 和 Pose 消息来分别控制小乌龟的运动和获取小乌龟的位置信息,实现了小乌龟画圆的功能。
阅读全文
相关推荐
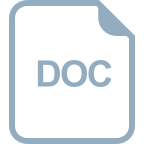
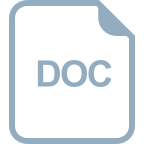
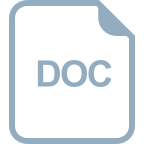















