拥有cv2.getPerspectiveTransform() 获得的透视变换矩阵后,怎么把原有坐标通过这个矩阵转化为矫正坐标
时间: 2024-03-05 07:54:42 浏览: 125
通过透视变换矩阵,可以将原始图像中的点映射到矫正后的图像中。具体步骤如下:
1. 使用cv2.getPerspectiveTransform()函数计算透视变换矩阵M,需要提供原始图像中的四个点和目标图像中对应的四个点。
2. 使用cv2.warpPerspective()函数将原始图像进行透视变换,得到矫正后的图像。
3. 对于需要转换的点P(x, y),将其表示为齐次坐标P' = [x, y, 1],然后通过矩阵乘法计算矫正后的点P'' = M * P'。
4. 对于矫正后的点P''(u, v, w),将其表示为非齐次坐标P(u/w, v/w)即为最终的矫正坐标。
示例代码如下:
```python
import cv2
import numpy as np
# 计算透视变换矩阵M
src_pts = np.float32([[0, 0], [0, 100], [100, 0], [100, 100]])
dst_pts = np.float32([[0, 0], [0, 200], [200, 0], [200, 200]])
M = cv2.getPerspectiveTransform(src_pts, dst_pts)
# 加载原始图像并进行透视变换
img = cv2.imread('test.jpg')
img_size = (img.shape[1], img.shape[0])
warped = cv2.warpPerspective(img, M, img_size)
# 转换需要矫正的点
x, y = 50, 50
P = np.float32([[x], [y], [1]])
P_ = np.dot(M, P)
u, v, w = P_[0], P_[1], P_[2]
x_, y_ = int(u/w), int(v/w)
print('矫正前坐标:({},{}),矫正后坐标:({},{})'.format(x, y, x_, y_))
```
其中,src_pts为原始图像中的四个点的坐标,dst_pts为目标图像中对应的四个点的坐标,M为透视变换矩阵。在转换需要矫正的点时,需要将其表示为齐次坐标P',然后通过矩阵乘法计算P'',最后将其表示为非齐次坐标即可得到矫正后的坐标。
阅读全文
相关推荐

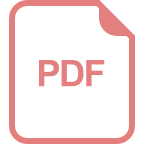
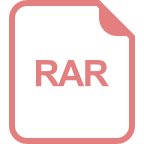
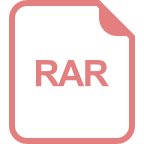
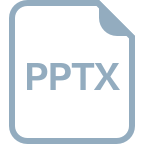
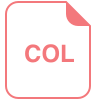
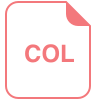
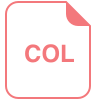







