BGR格式的图怎么用outputs_denoise = cv2.merge((outputs_denoise[:, :, 0], outputs_denoise[:, :, 1], outputs_denoise[:, :, 2]))
时间: 2024-01-18 07:03:29 浏览: 58
BGR格式的图像是一种常见的颜色空间,其中B表示蓝色通道,G表示绿色通道,R表示红色通道。在使用OpenCV进行图像处理时,经常需要将图像从其他颜色空间(例如RGB或灰度)转换为BGR格式。在将输出的图像显示或保存为图像文件时,通常需要将BGR格式的图像转换为RGB格式。
在上述代码中,使用了cv2.merge()函数将输出的图像的三个通道重新合并成一个BGR格式的图像。这里的参数是一个元组,包含三个分别表示蓝色、绿色和红色通道的NumPy数组。例如,outputs_denoise[:, :, 0]表示输出图像的蓝色通道,outputs_denoise[:, :, 1]表示输出图像的绿色通道,outputs_denoise[:, :, 2]表示输出图像的红色通道。通过将这三个通道合并,可以得到一张完整的BGR格式的图像。
需要注意的是,如果需要将BGR格式的图像转换为RGB格式,可以使用cv2.cvtColor()函数。例如,可以使用以下代码将BGR格式的图像转换为RGB格式:
```python
rgb_image = cv2.cvtColor(bgr_image, cv2.COLOR_BGR2RGB)
```
相关问题
import cv2 # 定义阈值(相似度百分比) threshold = 90 # 加载存储在闪存中的视频码流 flash_video = cv2.VideoCapture('path/to/flash_video.mp4') # 加载接收到的视频码流 received_video = cv2.VideoCapture('path/to/received_video.mp4') # 检查视频是否成功打开 if not flash_video.isOpened() or not received_video.isOpened(): print("无法打开视频文件") exit() frame_count = min(flash_video.get(cv2.CAP_PROP_FRAME_COUNT), received_video.get(cv2.CAP_PROP_FRAME_COUNT)) match_count = 0 for i in range(int(frame_count)): # 从闪存视频和接收到的视频中读取帧 ret_flash, frame_flash = flash_video.read() ret_received, frame_received = received_video.read() if not ret_flash or not ret_received: print("视频读取错误") break # 将帧转换为灰度图像进行比较 gray_flash = cv2.cvtColor(frame_flash, cv2.COLOR_BGR2GRAY) gray_received = cv2.cvtColor(frame_received, cv2.COLOR_BGR2GRAY) # 使用均方差计算帧之间的相似度 mse = ((gray_flash - gray_received) ** 2).mean() # 计算相似度百分比 similarity = (1 - mse / 255) * 100 # 判断帧是否相似 if similarity >= threshold: match_count += 1 # 计算相似度百分比 match_percentage = match_count / frame_count * 100 # 判断是否通过 if match_percentage >= threshold: print("通过") else: print("失败") # 释放资源 flash_video.release() received_video.release() 请将这段程序用C语言实现
以下是将上述Python代码转换为C语言的示例实现:
```c
#include <stdio.h>
#include <stdlib.h>
#include <opencv2/opencv.hpp>
#define THRESHOLD 90.0
int main() {
cv::VideoCapture flashVideo("path/to/flash_video.mp4");
cv::VideoCapture receivedVideo("path/to/received_video.mp4");
if (!flashVideo.isOpened() || !receivedVideo.isOpened()) {
printf("无法打开视频文件\n");
return -1;
}
double frameCount = std::min(flashVideo.get(cv::CAP_PROP_FRAME_COUNT), receivedVideo.get(cv::CAP_PROP_FRAME_COUNT));
int matchCount = 0;
for (int i = 0; i < frameCount; i++) {
cv::Mat frameFlash, frameReceived;
bool retFlash = flashVideo.read(frameFlash);
bool retReceived = receivedVideo.read(frameReceived);
if (!retFlash || !retReceived) {
printf("视频读取错误\n");
break;
}
cv::Mat grayFlash, grayReceived;
cv::cvtColor(frameFlash, grayFlash, cv::COLOR_BGR2GRAY);
cv::cvtColor(frameReceived, grayReceived, cv::COLOR_BGR2GRAY);
cv::Mat diff;
cv::absdiff(grayFlash, grayReceived, diff);
cv::Scalar mse = cv::mean(diff.mul(diff));
double similarity = (1.0 - mse.val[0] / 255.0) * 100.0;
if (similarity >= THRESHOLD) {
matchCount++;
}
}
double matchPercentage = matchCount / frameCount * 100.0;
if (matchPercentage >= THRESHOLD) {
printf("通过\n");
} else {
printf("失败\n");
}
flashVideo.release();
receivedVideo.release();
return 0;
}
```
请确保已经安装了OpenCV库,并根据你的环境进行必要的配置和链接。此示例代码将使用OpenCV库进行视频读取、图像处理和比较,实现了与Python代码相同的功能。如果需要进一步优化和适配,你可以根据自己的需求进行修改。希望对你有所帮助!
import cv2 import mediapipe as mp import time cap = cv2.VideoCapture(0) mpHands = mp.solutions.hands hands = mpHands.Hands() mpDraw = mp.solutions.drawing_utils pTime = 0 cTime = 0 while True: success, img = cap.read() imgRGB = cv2.cvtColor(img, cv2.COLOR_BGR2RGB) results = hands.process(imgRGB) #print(results.multi_hand_landmarks) if results.multi_hand_landmarks: for handLms in results.multi_hand_landmarks: for id, lm in enumerate(handLms.landmark): #print(id,lm) h, w, c = img.shape cx, cy = int(lm.x *w), int(lm.y*h) #if id ==0: cv2.circle(img, (cx,cy), 7, (255,0,255), cv2.FILLED) mpDraw.draw_landmarks(img, handLms, mpHands.HAND_CONNECTIONS) cTime = time.time() fps = 1/(cTime-pTime) pTime = cTime cv2.putText(img,str(int(fps)), (10,70), cv2.FONT_HERSHEY_PLAIN, 3, (255,0,255), 3) cv2.imshow("Image", img) cv2.waitKey(1)
这段代码使用OpenCV和MediaPipe库实现了手部姿势检测,并在视频流中绘制了手部关键点和连接线。具体实现如下:
1. 导入必要的库和模块:
```
import cv2
import mediapipe as mp
import time
```
2. 初始化摄像头并创建MediaPipe Hands模型:
```
cap = cv2.VideoCapture(0) # 初始化摄像头
mpHands = mp.solutions.hands # 创建MediaPipe Hands模型
hands = mpHands.Hands() # 实例化模型
mpDraw = mp.solutions.drawing_utils # 创建绘图工具
```
3. 循环读取视频帧并进行手部姿势检测:
```
while True:
success, img = cap.read() # 读取视频帧
imgRGB = cv2.cvtColor(img, cv2.COLOR_BGR2RGB) # 将BGR图像转换为RGB图像
results = hands.process(imgRGB) # 进行手部姿势检测
```
4. 绘制手部关键点和连接线:
```
if results.multi_hand_landmarks:
for handLms in results.multi_hand_landmarks:
for id, lm in enumerate(handLms.landmark):
h, w, c = img.shape
cx, cy = int(lm.x *w), int(lm.y*h)
cv2.circle(img, (cx,cy), 7, (255,0,255), cv2.FILLED)
mpDraw.draw_landmarks(img, handLms, mpHands.HAND_CONNECTIONS)
```
5. 计算并显示帧率:
```
cTime = time.time()
fps = 1/(cTime-pTime)
pTime = cTime
cv2.putText(img,str(int(fps)), (10,70), cv2.FONT_HERSHEY_PLAIN, 3, (255,0,255), 3)
```
6. 显示处理后的帧:
```
cv2.imshow("Image", img)
cv2.waitKey(1)
```
阅读全文
相关推荐
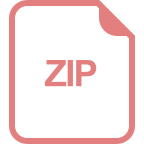
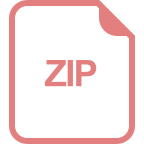
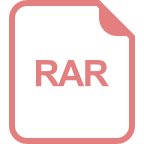














