import numpy as np def frame2mat(frameBuffer): """将帧信息转换为numpy的矩阵对象,后续可以通过opencv的cvtColor转换为特定的图像格式""" frame, buffer = frameBuffer bits = np.uint8 if (frame.bits == Bits.BITS_8) else np.uint16 shape = None if frame.format >= ImageFormat.FORMAT_MONO and frame.format <= ImageFormat.FORMAT_BAYER_RG: shape = 1 elif frame.format == ImageFormat.FORMAT_BGR24 or frame.format == ImageFormat.FORMAT_RGB24: shape = 3 elif frame.format == ImageFormat.FORMAT_BGR32 or frame.format == ImageFormat.FORMAT_RGB32: shape = 4 else: return None mat = np.ndarray(shape=(frame.iHeight, frame.iWidth, shape), dtype=bits, buffer=buffer) return mat帮我优化
时间: 2024-01-10 10:02:19 浏览: 121
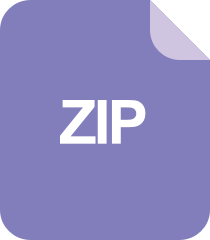
基于net的超市管理系统源代码(完整前后端+sqlserver+说明文档+LW).zip
可以对该函数进行以下优化:
1. 将np.uint8和np.uint16定义为常量,避免在函数调用时重复创建。
2. 通过字典存储格式信息和对应的shape,避免使用大量的if-else语句。
3. 将np.ndarray的shape参数修改为元组类型,避免在函数调用时创建新的元组对象。
修改后的代码如下:
```python
import numpy as np
UINT8 = np.uint8
UINT16 = np.uint16
SHAPE_DICT = {
ImageFormat.FORMAT_MONO: (1,),
ImageFormat.FORMAT_YUV422: (1,),
ImageFormat.FORMAT_BGR24: (3,),
ImageFormat.FORMAT_RGB24: (3,),
ImageFormat.FORMAT_BGR32: (4,),
ImageFormat.FORMAT_RGB32: (4,),
ImageFormat.FORMAT_BAYER_RG: (1,)
}
def frame2mat(frameBuffer):
"""将帧信息转换为numpy的矩阵对象,后续可以通过opencv的cvtColor转换为特定的图像格式"""
frame, buffer = frameBuffer
bits = UINT8 if frame.bits == Bits.BITS_8 else UINT16
shape = SHAPE_DICT.get(frame.format)
if shape is None:
return None
mat = np.ndarray(shape=(frame.iHeight, frame.iWidth, *shape), dtype=bits, buffer=buffer)
return mat
```
阅读全文
相关推荐
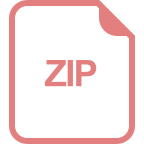