用蚁群算法求解tsp问题python
时间: 2024-12-12 16:13:34 浏览: 14
蚂蚁群优化(Ant Colony Optimization, ACO)是一种模拟生物群体行为的搜索算法,常用于解决旅行商问题(Travelling Salesman Problem, TSP)。在Python中,你可以使用像`deap`、`scipy`这样的库,以及自定义模拟蚂蚁的行为来求解TSP。
以下是简化的步骤:
1. **导入所需库**:安装并导入必要的库,如`numpy`, `networkx`, `deap`等。
```python
import numpy as np
from deap import base, creator, tools
import networkx as nx
```
2. **构建图模型**:创建一个表示城市之间距离的网络图。
```python
def create_graph(cities):
graph = nx.Graph()
for i in range(len(cities)):
for j in range(i+1, len(cities)):
graph.add_edge(i, j, weight=calculate_distance(cities[i], cities[j]))
return graph
```
3. **定义遗传算法组件**:包括个体(解)、适应度函数、选择、交叉和变异操作。
```python
creator.create("FitnessMax", base.Fitness, weights=(1.0,))
creator.create("Individual", list, fitness=creator.FitnessMax)
def tsp_fitness(individual, graph):
tour = [individual[0]]
for city in individual[1:]:
tour.append(city)
tour.append(tour[0]) # Close the tour
return nx.shortest_path_length(graph, source=tour[0], target=tour[-1], weight='weight')
toolbox = base.Toolbox()
toolbox.register("population", tools.initRepeat, list, creator.Individual)
toolbox.register("evaluate", tsp_fitness, graph=graph)
toolbox.register("mate", tools.cxTwoPoint)
toolbox.register("mutate", tools.mutShuffleIndexes, indpb=0.5 / len(graph.nodes))
```
4. **执行蚂蚁算法**:设置迭代次数,初始化种群,并通过循环执行选择、交叉和变异操作。
```python
def aco(num_ants, num_iterations):
pop = toolbox.population(n=num_ants)
h_values = compute_heuristic_values(graph)
for _ in range(num_iterations):
new_pop = []
for ant in pop:
new_ant = select_next_city(ant, graph, h_values)
new_pop.append(new_ant)
offspring = toolbox.select(new_pop, k=len(pop))
offspring = toolbox.mate(offspring)
offspring = toolbox.mutate(offspring)
offspring = [toolbox.evaluate(i) for i in offspring]
pop[:] = offspring
best_individual = min(pop, key=lambda ind: ind.fitness.values[0])
return best_individual
# ... 实现细节,比如计算启发式值、选择下一个城市等
```
阅读全文
相关推荐
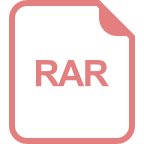
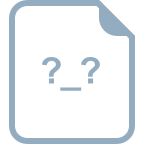
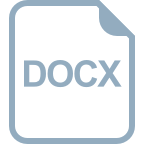
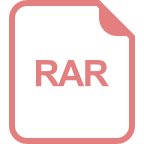
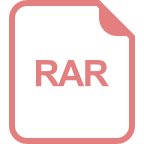
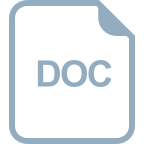
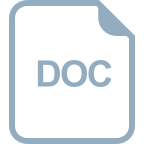
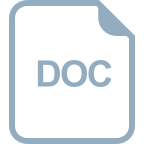







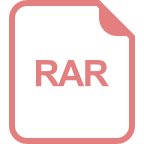
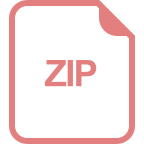
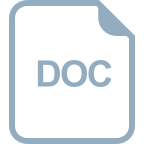