c++使用三文件方式,设计二维数据块类,数据成员包括数据块行数、列数、以及首地址;成员函数包括构造函数,求数据块最大值函数,数据块显示函数;主函数中,建立一个数据块对象并初始化,求最大值并显示最大值,显示数据块
时间: 2024-05-12 07:13:04 浏览: 12
首先,我们需要编写三个文件:头文件、实现文件和主函数文件。
1.头文件 Block.h
```cpp
#ifndef BLOCK_H
#define BLOCK_H
class Block
{
public:
Block(int rows, int cols, int* data);
int maxVal();
void display();
private:
int rows;
int cols;
int* data;
};
#endif
```
2.实现文件 Block.cpp
```cpp
#include "Block.h"
#include <iostream>
using namespace std;
Block::Block(int rows, int cols, int* data)
{
this->rows = rows;
this->cols = cols;
this->data = data;
}
int Block::maxVal()
{
int max = data[0];
for (int i = 1; i < rows * cols; i++)
{
if (data[i] > max)
max = data[i];
}
return max;
}
void Block::display()
{
for (int i = 0; i < rows; i++)
{
for (int j = 0; j < cols; j++)
{
cout << data[i * cols + j] << " ";
}
cout << endl;
}
}
```
3.主函数文件 main.cpp
```cpp
#include "Block.h"
#include <iostream>
using namespace std;
int main()
{
int rows = 3;
int cols = 4;
int data[] = { 1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11, 12 };
Block block(rows, cols, data);
block.display();
cout << "Max value: " << block.maxVal() << endl;
return 0;
}
```
在主函数中,我们创建了一个 Block 对象,并将其数据成员初始化为 3 行 4 列,数据为 {1,2,3,4,5,6,7,8,9,10,11,12}。然后调用对象的 display() 函数将其显示出来,再调用 maxVal() 函数求出最大值并输出。
编译并运行程序,可以得到如下输出:
```
1 2 3 4
5 6 7 8
9 10 11 12
Max value: 12
```
相关推荐
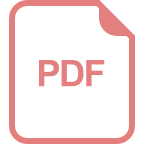
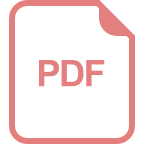
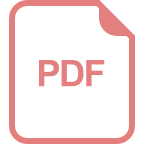














