Opengl基于qt显示照片 包括pro文件和头文件以及源文件
时间: 2024-01-19 22:03:10 浏览: 72
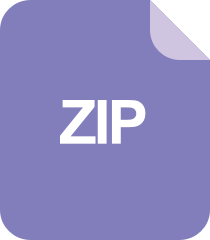
Opengl 相关的一些头文件和库文件

以下是基于 Qt 的 OpenGL 显示照片的代码示例,包括 .pro 文件、头文件和源文件:
1. .pro 文件:
```
QT += core gui opengl
greaterThan(QT_MAJOR_VERSION, 4): QT += widgets
TARGET = OpenGLPhotoViewer
TEMPLATE = app
# OpenGL 配置
CONFIG += opengl
LIBS += -lGL
SOURCES += \
main.cpp \
glwidget.cpp
HEADERS += \
glwidget.h
FORMS += \
mainwindow.ui
```
2. glwidget.h 头文件:
```cpp
#ifndef GLWIDGET_H
#define GLWIDGET_H
#include <QOpenGLWidget>
#include <QOpenGLFunctions>
#include <QOpenGLShaderProgram>
#include <QOpenGLTexture>
#include <QImage>
class GLWidget : public QOpenGLWidget, protected QOpenGLFunctions
{
public:
GLWidget(QWidget *parent = nullptr);
~GLWidget();
void setTextureImage(const QImage& image);
protected:
void initializeGL();
void resizeGL(int w, int h);
void paintGL();
private:
QOpenGLShaderProgram m_program;
QOpenGLTexture* m_texture = nullptr;
};
#endif // GLWIDGET_H
```
3. glwidget.cpp 源文件:
```cpp
#include "glwidget.h"
#include <QDebug>
GLWidget::GLWidget(QWidget *parent)
: QOpenGLWidget(parent)
{
}
GLWidget::~GLWidget()
{
makeCurrent();
delete m_texture;
doneCurrent();
}
void GLWidget::setTextureImage(const QImage &image)
{
makeCurrent();
// 创建纹理
if (!m_texture)
{
m_texture = new QOpenGLTexture(QOpenGLTexture::Target2D);
m_texture->setMinificationFilter(QOpenGLTexture::Linear);
m_texture->setMagnificationFilter(QOpenGLTexture::Linear);
m_texture->setWrapMode(QOpenGLTexture::ClampToEdge);
}
// 更新纹理数据
m_texture->setData(image);
doneCurrent();
}
void GLWidget::initializeGL()
{
initializeOpenGLFunctions();
// 加载顶点和片段着色器
m_program.addShaderFromSourceFile(QOpenGLShader::Vertex, ":/shaders/vertex.glsl");
m_program.addShaderFromSourceFile(QOpenGLShader::Fragment, ":/shaders/fragment.glsl");
m_program.link();
}
void GLWidget::resizeGL(int w, int h)
{
glViewport(0, 0, w, h);
}
void GLWidget::paintGL()
{
glClear(GL_COLOR_BUFFER_BIT);
// 绑定纹理
if (m_texture)
{
m_texture->bind();
}
// 绘制矩形
m_program.bind();
m_program.enableAttributeArray("vertexPosition");
m_program.setAttributeArray("vertexPosition", GL_FLOAT, nullptr, 2);
glDrawArrays(GL_TRIANGLE_FAN, 0, 4);
m_program.disableAttributeArray("vertexPosition");
m_program.release();
}
```
其中,vertex.glsl 和 fragment.glsl 是顶点和片段着色器文件,需要将它们放置在工程目录的 shaders 文件夹下:
vertex.glsl:
```glsl
attribute vec4 vertexPosition;
void main()
{
gl_Position = vertexPosition;
}
```
fragment.glsl:
```glsl
uniform sampler2D textureSampler;
void main()
{
gl_FragColor = texture2D(textureSampler, gl_TexCoord[0].st);
}
```
4. main.cpp 源文件:
```cpp
#include "mainwindow.h"
#include <QApplication>
int main(int argc, char *argv[])
{
QApplication a(argc, argv);
MainWindow w;
w.show();
return a.exec();
}
```
5. MainWindow.ui 文件:
在 Qt Designer 中创建一个 QWidget,将其转换成 QOpenGLWidget,然后将 GLWidget 实例添加到该 QOpenGLWidget 上。
以上就是基于 Qt 的 OpenGL 显示照片的代码示例,可以根据需要进行修改和完善。
阅读全文
相关推荐








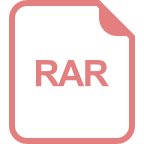
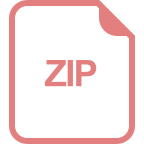
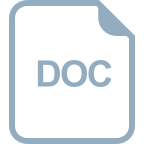


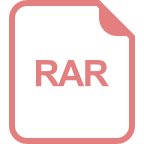
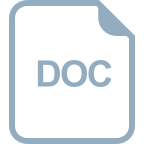
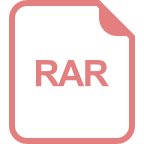