#ifndef _ASTAR_H #define _ASTAR_H #include <global_planner/planner_core.h> #include <global_planner/expander.h> #include <vector> #include <algorithm> namespace global_planner { class Index { public: Index(int a, float b) { i = a; cost = b; } int i; float cost; }; struct greater1 { bool operator()(const Index& a, const Index& b) const { return a.cost > b.cost; } }; class AStarExpansion : public Expander { public: AStarExpansion(PotentialCalculator* p_calc, int nx, int ny); virtual ~AStarExpansion() {} bool calculatePotentials(unsigned char* costs, double start_x, double start_y, double end_x, double end_y, int cycles, float* potential); private: void add(unsigned char* costs, float* potential, float prev_potential, int next_i, int end_x, int end_y); std::vector<Index> queue_; }; } //end namespace global_planner #endif
时间: 2024-04-18 09:30:53 浏览: 22
这是一个名为"AStarExpansion"的类的头文件,该类继承自"Expander"类,并实现了A*算法的路径规划功能。在命名空间"global_planner"中定义了该类。该类具有以下成员函数和成员变量:
成员函数:
- AStarExpansion:构造函数,接受一个PotentialCalculator指针对象和两个整数nx、ny作为参数。
- ~AStarExpansion:析构函数。
- calculatePotentials:计算路径规划的势能地图。接受一个unsigned char指针对象costs、四个double类型的起始点和终止点坐标、一个整数cycles和一个float类型指针对象potential作为参数。
成员变量:
- queue_:存储待扩展的节点的优先级队列。
另外,在代码中还定义了一个名为"Index"的类和一个名为"greater1"的结构体。
这段代码的作用是实现了A*算法的路径规划功能,并提供了计算势能地图的方法。
相关问题
#ifndef MY_HEADER_FILE_H #define MY_HEADER_FILE_H //#include "stdafx.h" #include <WINSOCK2.H> #include <iostream> #include <stdio.h> //#include <opencv/cv.h> #include <opencv2/core/core.hpp> #include <opencv2/highgui/highgui.hpp> #include <opencv2/imgproc/imgproc.hpp>
这段代码中的条件编译指令 `#ifndef` 和 `#define` 是正确的,但是在这个指令的下面,又出现了包含其他头文件的指令 `#include`。如果这些头文件中包含了当前头文件,就可能导致文件包含自身的问题。
为了避免这种情况,建议将所有的 `#include` 指令放在条件编译指令之间,例如:
```
#ifndef MY_HEADER_FILE_H
#define MY_HEADER_FILE_H
// 包含其他头文件
#include "stdafx.h"
#include <WINSOCK2.H>
#include <iostream>
#include <stdio.h>
#include <opencv/cv.h>
#include <opencv2/core/core.hpp>
#include <opencv2/highgui/highgui.hpp>
#include <opencv2/imgproc/imgproc.hpp>
// 头文件内容
#endif
```
这样,可以确保在条件编译指令之前包含的头文件不会包含当前头文件,避免了文件包含自身的问题。
#ifndef _PLANNER_MANAGER_H_ #define _PLANNER_MANAGER_H_ #include <stdlib.h> #include <bspline_opt/bspline_optimizer.h> #include <bspline_opt/uniform_bspline.h> #include <traj_utils/DataDisp.h> #include <plan_env/grid_map.h> #include <plan_env/obj_predic
这段代码是一个头文件 planner_manager.h 的内容。其中包含了一些头文件的引用,如 bspline_opt/bspline_optimizer.h、bspline_opt/uniform_bspline.h、traj_utils/DataDisp.h、plan_env/grid_map.h 和 plan_env/obj_predic。这些头文件提供了一些函数和数据类型,用于实现路径规划的相关功能,例如使用 B 样条优化器进行路径优化、使用 uniform B 样条进行插值、在地图中搜索路径、进行物体预测等等。如果你需要了解更详细的内容,可以查看这些头文件的具体实现。
相关推荐
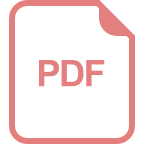












