写一个基于python的多元线性回归梯度算法
时间: 2024-04-30 07:22:33 浏览: 93
以下是基于Python的多元线性回归梯度算法:
```python
import numpy as np
class LinearRegression:
def __init__(self, learning_rate=0.01, num_iterations=1000):
self.learning_rate = learning_rate
self.num_iterations = num_iterations
self.weights = None
self.bias = None
def fit(self, X, y):
num_samples, num_features = X.shape
self.weights = np.zeros(num_features)
self.bias = 0
# Gradient descent
for i in range(self.num_iterations):
y_predicted = np.dot(X, self.weights) + self.bias
# Compute gradients
dw = (1 / num_samples) * np.dot(X.T, (y_predicted - y))
db = (1 / num_samples) * np.sum(y_predicted - y)
# Update weights and bias
self.weights -= self.learning_rate * dw
self.bias -= self.learning_rate * db
def predict(self, X):
y_predicted = np.dot(X, self.weights) + self.bias
return y_predicted
```
在这个算法中,我们首先定义了一个`LinearRegression`类,它有两个主要的参数:学习率和迭代次数。`fit`方法使用梯度下降算法拟合模型。它首先初始化权重和偏置,然后在每次迭代中计算预测输出和梯度,并使用它们更新权重和偏置。`predict`方法使用训练后的模型进行预测。它首先计算预测输出,然后返回它们。
使用这个算法进行多元线性回归,我们需要将训练数据和标签传递给`fit`方法,然后使用`predict`方法进行预测。以下是一个例子:
```python
# Generate sample data
num_samples = 100
num_features = 3
X = np.random.randn(num_samples, num_features)
y = np.random.randn(num_samples)
# Train the model
lr = LinearRegression()
lr.fit(X, y)
# Make predictions
X_test = np.random.randn(10, num_features)
y_pred = lr.predict(X_test)
print(y_pred)
```
在这个例子中,我们生成了100个样本,每个样本有3个特征。我们使用随机数生成这些样本。然后我们生成了100个随机标签。我们使用这些数据训练了线性回归模型,并使用它对10个新样本进行了预测。最后,我们打印了这些预测输出。
阅读全文
相关推荐






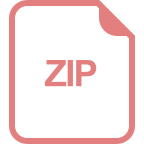
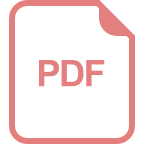
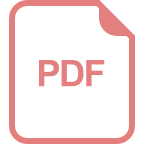
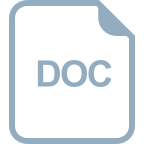




