实现一个子进程向一消息队列发送一段字符串,另一子进程从该消息队列出取出该消息, 并输出到标准输出。
时间: 2024-04-30 09:22:06 浏览: 11
可以使用System V IPC机制中的消息队列实现。子进程1使用msgsnd函数向消息队列发送消息,子进程2使用msgrcv函数从消息队列中接收消息,并输出到标准输出。注意要对消息队列进行初始化和清理。以下是代码示例:
子进程1:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <sys/ipc.h>
#include <sys/msg.h>
#define MSG_SIZE 1024
struct msgbuf {
long mtype;
char mtext[MSG_SIZE];
};
int main() {
key_t key;
int msgid;
struct msgbuf buf;
// 创建消息队列
key = ftok(".", 'a');
if (key == -1) {
perror("ftok");
exit(1);
}
msgid = msgget(key, IPC_CREAT | 0666);
if (msgid == -1) {
perror("msgget");
exit(1);
}
// 发送消息
buf.mtype = 1;
strcpy(buf.mtext, "Hello, world!");
if (msgsnd(msgid, &buf, strlen(buf.mtext) + 1, 0) == -1) {
perror("msgsnd");
exit(1);
}
return 0;
}
```
子进程2:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <sys/ipc.h>
#include <sys/msg.h>
#define MSG_SIZE 1024
struct msgbuf {
long mtype;
char mtext[MSG_SIZE];
};
int main() {
key_t key;
int msgid;
struct msgbuf buf;
// 获取消息队列
key = ftok(".", 'a');
if (key == -1) {
perror("ftok");
exit(1);
}
msgid = msgget(key, 0666);
if (msgid == -1) {
perror("msgget");
exit(1);
}
// 接收消息
if (msgrcv(msgid, &buf, MSG_SIZE, 1, 0) == -1) {
perror("msgrcv");
exit(1);
}
printf("%s\n", buf.mtext);
// 删除消息队列
if (msgctl(msgid, IPC_RMID, NULL) == -1) {
perror("msgctl");
exit(1);
}
return 0;
}
```
相关推荐
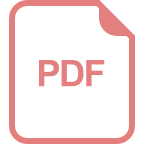
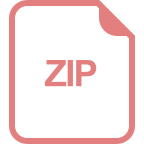
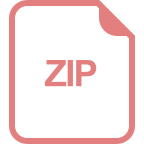














