Miller-Rabin素性检验算法C语言
时间: 2023-07-10 10:39:13 浏览: 131
Miller-Rabin 素性检验算法是一种基于随机化的素性检验算法。下面是一个用 C 语言实现的 Miller-Rabin 素性检验算法的示例代码:
```
#include <stdio.h>
#include <stdlib.h>
#include <time.h>
#include <math.h>
int modular_exponentiation(int base, int exponent, int modulus)
{
int result = 1;
while (exponent > 0)
{
if (exponent % 2 == 1)
{
result = (result * base) % modulus;
}
base = (base * base) % modulus;
exponent = exponent / 2;
}
return result;
}
int miller_rabin(int n, int k)
{
if (n <= 1 || n == 4)
{
return 0;
}
if (n <= 3)
{
return 1;
}
int d = n - 1;
while (d % 2 == 0)
{
d = d / 2;
}
for (int i = 0; i < k; i++)
{
int a = 2 + rand() % (n - 3);
int x = modular_exponentiation(a, d, n);
if (x == 1 || x == n - 1)
{
continue;
}
int j;
for (j = 0; j < (int)log2(n - 1); j++)
{
x = (x * x) % n;
if (x == 1)
{
return 0;
}
if (x == n - 1)
{
break;
}
}
if (j == (int)log2(n - 1))
{
return 0;
}
}
return 1;
}
int main()
{
srand(time(NULL));
int n, k;
printf("Enter a number to check if it is prime: ");
scanf("%d", &n);
printf("Enter the number of iterations for the test: ");
scanf("%d", &k);
if (miller_rabin(n, k))
{
printf("%d is probably prime.\n", n);
}
else
{
printf("%d is composite.\n", n);
}
return 0;
}
```
这个程序中,`modular_exponentiation` 函数用于计算模幂,`miller_rabin` 函数用于执行 Miller-Rabin 素性检验。在 `main` 函数中,用户输入要检验的数和测试的迭代次数,然后调用 `miller_rabin` 函数进行素性检验。最终输出结果。
相关推荐
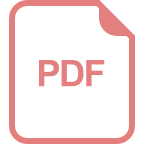
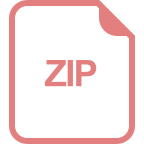
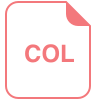
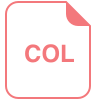
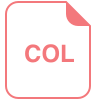
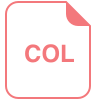
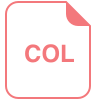








