import osimport reimport pandas as pd# 正则表达式regex = re.compile(r'^2-.*\.xlsx$')continuation_regex = re.compile(r'^\d+\s.*$') # 这里假设续表的第一列为数字加空格# 读取所有符合条件的xlsx文件files = [f for f in os.listdir('.') if os.path.isfile(f) and regex.match(f)]dfs = []for file in files: df = pd.read_excel(file, sheet_name=None, engine='openpyxl') for sheet_name, sheet_df in df.items(): # 判断续表并添加到之前的表格中 if sheet_df.iloc[0, 0] and continuation_regex.match(str(sheet_df.iloc[0, 0])): dfs[-1] = pd.concat([dfs[-1], sheet_df]) else: dfs.append(sheet_df)# 合并所有表格merged_df = pd.concat(dfs)# 根据城市为键合并所有表格grouped_df = merged_df.groupby('城市').agg(lambda x: x.tolist())# 将所有列表转换为字符串并重新转换为DataFramegrouped_df = grouped_df.applymap(lambda x: ','.join([str(i) for i in x]))grouped_df = pd.DataFrame(grouped_df.to_dict())# 输出结果print(grouped_df) 修改为遍历指定列表
时间: 2024-02-23 10:02:58 浏览: 25
如果想要修改这段代码,使其不再遍历当前文件夹下的xlsx文件,而是遍历指定文件夹下的xlsx文件,可以将os.listdir('.')替换为os.listdir('指定文件夹路径'),例如:
```
import os
import re
import pandas as pd
# 正则表达式
regex = re.compile(r'^2-.*\.xlsx$')
continuation_regex = re.compile(r'^\d+\s.*$') # 这里假设续表的第一列为数字加空格
# 指定文件夹路径
folder_path = '指定文件夹路径'
# 读取所有符合条件的xlsx文件
files = [os.path.join(folder_path, f) for f in os.listdir(folder_path) if os.path.isfile(os.path.join(folder_path, f)) and regex.match(f)]
dfs = []
for file in files:
df = pd.read_excel(file, sheet_name=None, engine='openpyxl')
for sheet_name, sheet_df in df.items():
# 判断续表并添加到之前的表格中
if sheet_df.iloc[0, 0] and continuation_regex.match(str(sheet_df.iloc[0, 0])):
dfs[-1] = pd.concat([dfs[-1], sheet_df])
else:
dfs.append(sheet_df)
# 合并所有表格
merged_df = pd.concat(dfs)
# 根据城市为键合并所有表格
grouped_df = merged_df.groupby('城市').agg(lambda x: x.tolist())
# 将所有列表转换为字符串并重新转换为DataFrame
grouped_df = grouped_df.applymap(lambda x: ','.join([str(i) for i in x]))
grouped_df = pd.DataFrame(grouped_df.to_dict())
# 输出结果
print(grouped_df)
```
其中,需要将'指定文件夹路径'替换为实际的文件夹路径。
相关推荐
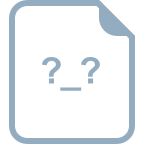
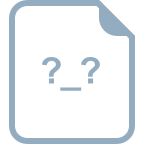














