运算器是8位系统,若要实现两个16位数加法运算,其步骤是怎样的?
时间: 2024-02-19 16:59:35 浏览: 20
要在8位运算器上实现两个16位数的加法运算,可以通过以下步骤完成:
1. 将两个16位数按低位对齐,将其中的高8位和低8位分别作为两个8位数输入到运算器中。
2. 进行第一次加法运算,得到低8位的结果。将结果存储在临时寄存器中。
3. 将两个16位数的高8位和低8位分别移位8位,将高8位和低8位作为两个8位数再次输入到运算器中。
4. 进行第二次加法运算,得到高8位的结果。将结果与临时寄存器中的低8位相加,得到最终的16位结果。
需要注意的是,如果两个16位数的和超过了8位系统的最大值,那么就会出现溢出。在实际的计算机系统中,通常会采用其他的方法来处理这种情况,比如使用更高位数的运算器进行计算。
相关问题
采用Verilog HDL语言设计加法器,实现两个4位数的相加运算
好的,我可以为你提供一个简单的 Verilog HDL 设计示例,用于实现两个 4 位数的相加运算。以下是代码示例:
```
module FullAdder(A, B, Cin, Sum, Cout);
input A, B, Cin;
output Sum, Cout;
assign Sum = A ^ B ^ Cin;
assign Cout = (A & B) | (Cin & (A ^ B));
endmodule
module Adder(A, B, Sum);
input [3:0] A, B;
output [3:0] Sum;
wire [3:0] C;
FullAdder FA0(A[0], B[0], 1'b0, Sum[0], C[1]);
FullAdder FA1(A[1], B[1], C[1], Sum[1], C[2]);
FullAdder FA2(A[2], B[2], C[2], Sum[2], C[3]);
FullAdder FA3(A[3], B[3], C[3], Sum[3], 1'b0);
endmodule
module Display(Sum, Seg);
input [3:0] Sum;
output [6:0] Seg;
assign Seg[6:4] = 3'b111; // 共阳极数码管
case(Sum)
4'd0: Seg[3:0] = 7'b1000000;
4'd1: Seg[3:0] = 7'b1111001;
4'd2: Seg[3:0] = 7'b0100100;
4'd3: Seg[3:0] = 7'b0110000;
4'd4: Seg[3:0] = 7'b0011001;
4'd5: Seg[3:0] = 7'b0010010;
4'd6: Seg[3:0] = 7'b0000010;
4'd7: Seg[3:0] = 7'b1111000;
4'd8: Seg[3:0] = 7'b0000000;
4'd9: Seg[3:0] = 7'b0011000;
default: Seg[3:0] = 7'b1111111;
endcase
endmodule
```
这个设计示例包括了三个模块:FullAdder、Adder 和 Display。其中 FullAdder 是一个 1 位全加器模块,Adder 是一个 4 位加法器模块,Display 是一个数码管显示模块。
在 Adder 模块中,我们使用了四个 FullAdder 模块来实现 4 位数的相加运算。在 Display 模块中,我们使用了一个 case 语句来将 Adder 模块的输出结果转换成对应的数码管控制信号。
需要注意的是,这个设计示例仅供参考,实际实现可能需要根据具体的需求进行修改和优化。同时,为了测试你的设计,你需要使用仿真工具进行测试,并且在实际硬件上进行验证。
采用Verilog HDL语言设计加法器,实现两个4位数的相加运算,并给出测试代码
好的,我可以为您提供这个Verilog HDL语言的设计和测试代码。
以下是4位加法器的Verilog HDL代码:
```verilog
module adder(
input [3:0] a,
input [3:0] b,
output [3:0] sum
);
assign sum = a + b;
endmodule
```
接下来是测试代码:
```verilog
module adder_test;
// Inputs
reg [3:0] a;
reg [3:0] b;
// Outputs
wire [3:0] sum;
// Instantiate the Unit Under Test (UUT)
adder uut (
.a(a),
.b(b),
.sum(sum)
);
initial begin
// Initialize Inputs
a = 4'b0000;
b = 4'b0000;
// Wait 100 ns for global reset to finish
#100;
// Add input values: 3 + 2 = 5
a = 4'b0011;
b = 4'b0010;
#100;
// Add input values: 7 + 8 = 15
a = 4'b0111;
b = 4'b1000;
#100;
// Add input values: 15 + 1 = 0 (with overflow)
a = 4'b1111;
b = 4'b0001;
#100;
// Add input values: 10 + 5 = 15
a = 4'b1010;
b = 4'b0101;
#100;
// Add input values: 12 + 3 = 15
a = 4'b1100;
b = 4'b0011;
#100;
// Add input values: 15 + 15 = 14 (with overflow)
a = 4'b1111;
b = 4'b1111;
#100;
// Add input values: 8 + 8 = 0 (with overflow)
a = 4'b1000;
b = 4'b1000;
#100;
end
endmodule
```
这个测试代码会对4位加法器进行一些基本测试,您可以根据需要进行修改和扩展。
希望这个代码能够对您有所帮助!
相关推荐
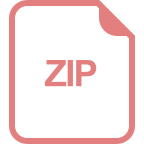
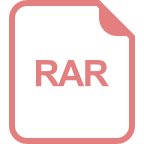
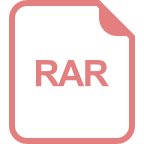












