贪心算法最优装载问题c语言代码
时间: 2024-11-19 21:15:43 浏览: 54
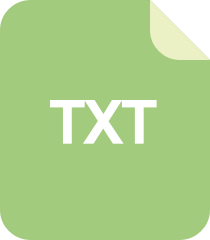
最优装载问题(贪心算法)c++

贪心算法在解决最优装载问题时,通常用于寻找给定物品集合在限制条件下能够达到的最大价值组合。在这个场景下,例如我们要找一个背包能装下的最大价值物品,一种常见的贪心策略是每次选择当前剩余容量内价值最大的物品。
以下是一个简单的C语言代码示例,用贪心算法解决0-1背包问题(每件物品只能取一次):
```c
#include <stdio.h>
// 定义物品结构体
typedef struct {
int weight; // 物品重量
int value; // 物品价值
} Item;
// 比较函数,判断是否应该优先选择当前物品
int compare(const void *a, const void *b) {
Item *itemA = (Item *)a;
Item *itemB = (Item *)b;
if (itemA->value > itemB->value)
return -1;
else if (itemA->value < itemB->value)
return 1;
else
return itemA->weight - itemB->weight; // 如果价值相等,按重量小的优先
}
// 贪心填充函数
void greedyKnapsack(Item items[], int n, int capacity) {
qsort(items, n, sizeof(Item), compare); // 对物品进行排序
for (int i = 0; i < n && items[i].weight <= capacity; i++) {
if (capacity >= items[i].weight) {
capacity -= items[i].weight;
printf("Picked item %d with weight %d and value %d\n", i + 1, items[i].weight, items[i].value);
} else {
printf("Picked item %d with remaining capacity %d\n", i + 1, capacity);
break;
}
}
}
int main() {
Item items[] = { {10, 60}, {20, 100}, {30, 120}, {40, 150} };
int n = sizeof(items) / sizeof(items[0]);
int capacity = 50;
greedyKnapsack(items, n, capacity);
return 0;
}
```
这个程序首先对物品按照价值降序或升序加重量排序,然后从价值最高的开始尝试放入背包,直到无法再装或者背包已满。
阅读全文
相关推荐
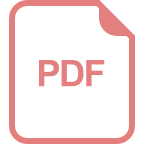
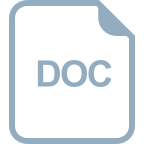









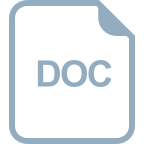





