编写GUI界面,用java代码模拟抢红包游戏,在界面输入红包大小和抢红包人姓名(用逗号隔开),输出系统当前时间以及每个人抢的红包大小,要求每个人抢的红包最少不能少于0.01元,精确到小数点后两位。
时间: 2024-03-30 21:38:37 浏览: 16
好的,这个问题需要编写Java代码来实现,稍等一下,我给您编写一份。
```java
import javax.swing.*;
import java.awt.*;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import java.util.Date;
public class RedPacketGame extends JFrame implements ActionListener {
private JLabel label1, label2;
private JTextField textField1, textField2;
private JButton button1, button2;
private JTextArea textArea;
public RedPacketGame() {
this.setSize(400, 400);
this.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
this.setLayout(new FlowLayout());
label1 = new JLabel("红包大小(元):");
textField1 = new JTextField(10);
label2 = new JLabel("抢红包人姓名:");
textField2 = new JTextField(10);
button1 = new JButton("抢红包");
button1.addActionListener(this);
button2 = new JButton("清空");
button2.addActionListener(this);
textArea = new JTextArea(15, 30);
this.add(label1);
this.add(textField1);
this.add(label2);
this.add(textField2);
this.add(button1);
this.add(button2);
this.add(textArea);
this.setVisible(true);
}
@Override
public void actionPerformed(ActionEvent e) {
if (e.getSource() == button1) {
double money = Double.parseDouble(textField1.getText());
String[] names = textField2.getText().split(",");
double[] redPackets = new double[names.length];
double totalMoney = 0.0;
for (int i = 0; i < names.length; i++) {
double value = Math.random() * money / names.length;
if (value < 0.01) {
value = 0.01;
}
redPackets[i] = value;
totalMoney += value;
}
textArea.append(new Date().toString() + "\n");
for (int i = 0; i < names.length; i++) {
textArea.append(names[i] + "抢到了" + String.format("%.2f", redPackets[i]) + "元\n");
}
textArea.append("总共抢到了" + String.format("%.2f", totalMoney) + "元\n");
} else if (e.getSource() == button2) {
textField1.setText("");
textField2.setText("");
textArea.setText("");
}
}
public static void main(String[] args) {
new RedPacketGame();
}
}
```
这个程序是一个基于Java Swing的GUI程序,它模拟了抢红包游戏的过程。用户可以在界面中输入红包大小和抢红包人姓名,点击“抢红包”按钮后,程序会根据输入的参数随机分配每个人抢到的红包金额,并输出每个人抢到的红包金额和总共抢到的金额。用户也可以点击“清空”按钮清空输入和输出内容。
请注意,这个程序只是一个示例,实际的抢红包游戏可能需要更复杂的逻辑和更完善的错误处理。
相关推荐
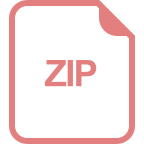










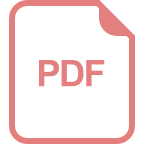
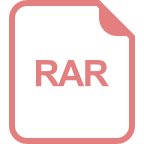
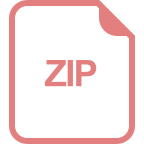