a3c代码
时间: 2023-07-02 22:19:06 浏览: 112
以下是一个简单的 A3C 实现代码,仅供参考:
```python
import gym
import numpy as np
import tensorflow as tf
import threading
global_episode = 0
global_rewards = []
global_episodes = 10000
episode_rewards = tf.keras.metrics.Mean('episode_rewards', dtype=tf.float32)
class A3C(tf.keras.Model):
def __init__(self, state_size, action_size):
super(A3C, self).__init__()
self.state_size = state_size
self.action_size = action_size
self.dense1 = tf.keras.layers.Dense(64, activation='relu')
self.dense2 = tf.keras.layers.Dense(64, activation='relu')
self.policy_logits = tf.keras.layers.Dense(action_size)
self.values = tf.keras.layers.Dense(1)
def call(self, inputs):
x = self.dense1(inputs)
x = self.dense2(x)
logits = self.policy_logits(x)
values = self.values(x)
return logits, values
class Agent:
def __init__(self, state_size, action_size):
self.state_size = state_size
self.action_size = action_size
self.global_model = A3C(state_size, action_size)
self.global_model(tf.keras.Input(shape=(state_size,)))
self.opt = tf.optimizers.Adam(learning_rate=.0001, clipnorm=1.0)
self.gamma = 0.99
self.tau = .125
def train(self, state, action, reward, next_state, done):
with tf.GradientTape() as tape:
logits, value = self.global_model(tf.convert_to_tensor(state[None, :], dtype=tf.float32))
next_logits, next_value = self.global_model(tf.convert_to_tensor(next_state[None, :], dtype=tf.float32))
advantage = reward + self.gamma * next_value[0] * (1 - int(done)) - value[0]
value_loss = advantage ** 2
policy = tf.nn.softmax(logits)
entropy = tf.reduce_sum(policy * tf.math.log(policy))
policy_loss = tf.nn.sparse_softmax_cross_entropy_with_logits(labels=action, logits=logits)
total_loss = tf.reduce_mean(.5 * value_loss + policy_loss - .01 * entropy)
grads = tape.gradient(total_loss, self.global_model.trainable_variables)
self.opt.apply_gradients(zip(grads, self.global_model.trainable_variables))
def get_action(self, state):
logits, _ = self.global_model(tf.convert_to_tensor(state[None, :], dtype=tf.float32))
probs = tf.nn.softmax(logits)
action = np.random.choice(self.action_size, p=probs.numpy()[0])
return action
def sync(self, local_model):
for local, global_ in zip(local_model.trainable_variables, self.global_model.trainable_variables):
global_.assign(self.tau * local + (1 - self.tau) * global_)
def test(env, agent):
state = env.reset()
done = False
total_reward = 0
while not done:
action = agent.get_action(state)
next_state, reward, done, _ = env.step(action)
state = next_state
total_reward += reward
return total_reward
def train(global_agent, num_episodes, lock):
global global_episode, global_rewards
env = gym.make('CartPole-v0')
agent = Agent(env.observation_space.shape[0], env.action_space.n)
for ep in range(num_episodes):
state = env.reset()
done = False
episode_reward = 0
while not done:
action = agent.get_action(state)
next_state, reward, done, _ = env.step(action)
agent.train(state, action, reward, next_state, done)
state = next_state
episode_reward += reward
with lock:
global_rewards.append(episode_reward)
global_episode += 1
episode_rewards(episode_reward)
print("Episode: {}, Reward: {}".format(global_episode, episode_reward))
agent.sync(agent)
if global_episode % 100 == 0:
test_reward = test(env, agent)
print("Test Reward: {}".format(test_reward))
if __name__ == '__main__':
lock = threading.Lock()
global_agent = Agent(4, 2)
threads = []
for i in range(4):
t = threading.Thread(target=train, args=(global_agent, global_episodes//4, lock))
threads.append(t)
for thread in threads:
thread.start()
for thread in threads:
thread.join()
```
在这个实现中,我们首先定义了一个 A3C 模型和一个 Agent 类,其中 A3C 模型有两个输出:一个是策略 logits,一个是状态值估计。Agent 类负责在环境中与模型进行交互,以及使用梯度下降更新模型。
我们使用了一个简单的 CartPole 环境来测试模型。在训练过程中,我们创建了四个线程来并行地训练模型,每个线程都有自己的 local 模型。每个 episode 结束时,local 模型的参数会同步到 global 模型中。
此外,我们还定义了一个 test 函数来测试模型的性能。在每个训练周期的末尾,我们都会调用这个函数来评估模型在测试集上的表现。
请注意,这只是一个简单的实现,无法保证在所有环境中都能正常运行。如果你想要在自己的项目中使用 A3C,建议参考一些开源的实现,如 Tensorflow 的官方实现。
阅读全文
相关推荐
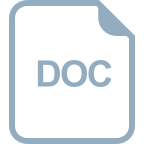
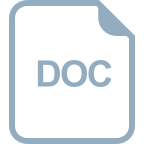
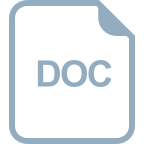








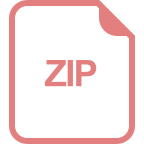
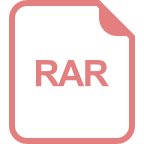
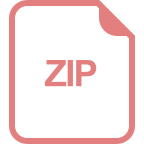
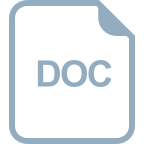
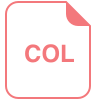
