python 遗传算法 sklearn
时间: 2023-09-04 07:02:00 浏览: 139
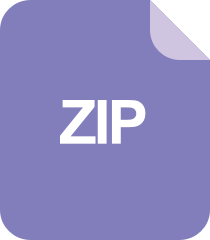
使用受 scikit-learn 启发的 API 使用遗传 算法 构建决策树_python_代码_下载
Python遗传算法的库中,sklearn是一个非常流行和广泛使用的机器学习库。遗传算法是一种基于生物进化思想的优化算法,可以应用于很多机器学习问题中。Sklearn提供了一些用于构建遗传算法的工具和函数。
在sklearn中,可以使用遗传算法来解决参数优化问题。例如,如果我们想要找到一个机器学习模型的最佳参数组合,可以使用遗传算法来对参数进行搜索。首先,我们定义一个适应度函数,用于评估每个参数组合的性能。然后,我们使用遗传算法的运算符,如选择、交叉和变异,对参数进行进化操作。最终,遗传算法会为我们找到最佳参数组合。
在sklearn中,我们可以使用遗传算法的工具和函数来实现上述步骤。例如,可以使用sklearn的cross_val_score函数作为适应度函数,用于评估每个参数组合的性能。然后,可以使用sklearn的遗传算法工具箱进行选择、交叉和变异操作。最后,可以使用遗传算法的工具函数,如fit和predict,来应用找到的最佳参数组合,构建机器学习模型,并对新数据进行预测。
总的来说,sklearn是一个用于机器学习的Python库,可以与遗传算法一起使用。通过使用sklearn中的工具和函数,我们可以方便地构建和应用遗传算法来解决各种优化问题,包括机器学习模型的参数优化。
阅读全文
相关推荐
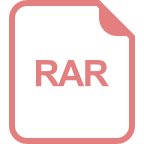
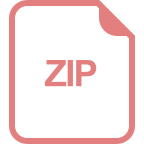
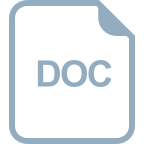
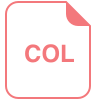
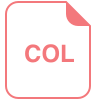










