在M031KG系列里,,用UART_SetTimeoutCnt函数,实现UART1串口接收超时中断
时间: 2024-02-20 21:58:21 浏览: 211
在M031KG系列微控制器中,可以使用UART_SetTimeoutCnt函数来设置UART串口接收超时时间,并实现超时中断。下面是实现UART1串口接收超时中断的步骤:
1. 首先需要初始化UART1串口,可以使用UART_Open函数进行初始化,设置相应的波特率、数据位、停止位、校验位等参数。
2. 然后使用UART_SetTimeoutCnt函数设置UART1串口接收超时时间,该函数的第一个参数为UART端口号,这里设置为UART_PORT1;第二个参数为超时计数器,即当接收到一个字符后,计数器从该值开始倒计时,如果在倒计时过程中没有再接收到字符,则会触发超时中断;第三个参数为超时中断使能,这里设置为使能。
3. 最后需要配置UART1串口接收中断,使能接收中断和超时中断。可以使用NVIC_EnableIRQ函数使能UART1中断,并在中断服务函数中处理接收数据和超时中断。
下面是实现UART1串口接收超时中断的示例代码:
```
#include "stdio.h"
#include "NuMicro.h"
#define RXBUFSIZE 1024
volatile uint8_t comRbuf[RXBUFSIZE];
volatile uint16_t comRbytes = 0; // Available bytes of comRbuf
volatile uint16_t comRhead = 0;
volatile uint16_t comRtail = 0;
void UART1_IRQHandler(void)
{
uint32_t u32IntSts = UART1->INTSTS;
if(u32IntSts & UART_INTSTS_RDAIF_Msk)
{
/* Get all the input characters */
while(UART_IS_RX_READY(UART1))
{
/* Check if buffer full */
if(comRbytes < RXBUFSIZE)
{
/* Enqueue the character */
comRbuf[comRtail++] = UART_READ(UART1);
if(comRtail >= RXBUFSIZE)
comRtail = 0;
comRbytes++;
}
else
{
/* FIFO over run */
}
}
}
if(u32IntSts & UART_INTSTS_RXTOIF_Msk)
{
/* Timeout counter flag */
/* Reset FIFO */
UART1->FIFO &= ~UART_FIFO_RXRST_Msk;
/* Disable RX time-out interrupt */
UART_DisableInt(UART1, UART_INTEN_RXTOIEN_Msk);
/* Clear RX time-out interrupt flag */
UART1->INTSTS = UART_INTSTS_RXTOIF_Msk;
}
}
int32_t main (void)
{
uint32_t u32data;
/* Unlock protected registers */
SYS_UnlockReg();
/* Enable UART1 peripheral clock */
CLK_EnableModuleClock(UART1_MODULE);
/* Select UART1 module function */
SYS->GPA_MFPH = (SYS->GPA_MFPH & (~SYS_GPA_MFPH_PA10MFP_Msk)) | SYS_GPA_MFPH_PA10MFP_UART1_RXD;
SYS->GPA_MFPH = (SYS->GPA_MFPH & (~SYS_GPA_MFPH_PA9MFP_Msk)) | SYS_GPA_MFPH_PA9MFP_UART1_TXD;
/* Disable UART1 TX/RX function */
UART1->FUNCSEL = (UART1->FUNCSEL & (~(UART_FUNCSEL_RXDIS_Msk | UART_FUNCSEL_TXDIS_Msk)));
/* Set UART1 line configuration */
UART_Open(UART1, 115200);
/* Enable UART1 RX time-out interrupt */
UART_SetTimeoutCnt(UART1, 40);
UART_EnableInt(UART1, UART_INTEN_RXTOIEN_Msk);
NVIC_EnableIRQ(UART1_IRQn);
while(1)
{
/* Get all the input characters */
while(comRbytes)
{
/* Get a character from the buffer */
u32data = comRbuf[comRhead++];
if(comRhead >= RXBUFSIZE)
comRhead = 0;
comRbytes--;
printf("Received: 0x%02X\n", u32data);
}
}
}
```
在上面的示例代码中,设置UART1的超时计数器为40,即当接收到一个字符后,计数器从40开始倒计时,如果在倒计时过程中没有再接收到字符,则会触发超时中断。中断服务函数中处理接收到的字符和超时中断,在接收到字符时将其存入循环队列中,超时中断时禁用超时中断并清除超时中断标志位。在主函数中循环读取循环队列中的字符并打印。
阅读全文
相关推荐
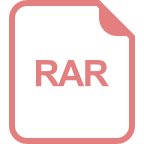
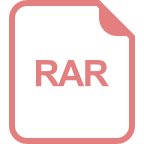
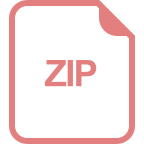















