用C语言写栈实现四则运算的代码
时间: 2024-05-10 20:20:28 浏览: 98
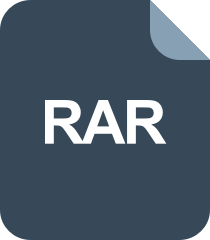
四则运算,C语言栈实现.rar
以下是使用C语言实现栈实现四则运算的代码:
```c
#include <stdio.h>
#include <stdlib.h>
#define MAX_SIZE 100
#define ERROR -2147483648
typedef struct {
int top;
int data[MAX_SIZE];
} Stack;
void initStack(Stack *s) {
s->top = -1;
}
int isEmpty(Stack *s) {
return s->top == -1;
}
int isFull(Stack *s) {
return s->top == MAX_SIZE - 1;
}
void push(Stack *s, int x) {
if (isFull(s)) {
printf("Error: stack is full\n");
return;
}
s->data[++s->top] = x;
}
int pop(Stack *s) {
if (isEmpty(s)) {
printf("Error: stack is empty\n");
return ERROR;
}
return s->data[s->top--];
}
int peek(Stack *s) {
if (isEmpty(s)) {
printf("Error: stack is empty\n");
return ERROR;
}
return s->data[s->top];
}
int priority(char c) {
if (c == '+' || c == '-') {
return 1;
} else if (c == '*' || c == '/') {
return 2;
} else {
return 0;
}
}
int calc(int a, int b, char op) {
if (op == '+') {
return a + b;
} else if (op == '-') {
return a - b;
} else if (op == '*') {
return a * b;
} else if (op == '/') {
return a / b;
} else {
printf("Error: invalid operator\n");
return ERROR;
}
}
int evaluate(char *expr) {
Stack numStack, opStack;
initStack(&numStack);
initStack(&opStack);
int i = 0;
while (expr[i] != '\0') {
if (expr[i] >= '0' && expr[i] <= '9') {
int num = 0;
while (expr[i] >= '0' && expr[i] <= '9') {
num = num * 10 + (expr[i] - '0');
i++;
}
push(&numStack, num);
} else if (expr[i] == '+' || expr[i] == '-' || expr[i] == '*' || expr[i] == '/') {
while (!isEmpty(&opStack) && priority(peek(&opStack)) >= priority(expr[i])) {
int b = pop(&numStack);
int a = pop(&numStack);
char op = pop(&opStack);
int result = calc(a, b, op);
push(&numStack, result);
}
push(&opStack, expr[i]);
i++;
} else {
printf("Error: invalid character\n");
return ERROR;
}
}
while (!isEmpty(&opStack)) {
int b = pop(&numStack);
int a = pop(&numStack);
char op = pop(&opStack);
int result = calc(a, b, op);
push(&numStack, result);
}
return pop(&numStack);
}
int main() {
char expr[MAX_SIZE];
printf("Please enter the expression: ");
scanf("%s", expr);
int result = evaluate(expr);
printf("Result = %d\n", result);
return 0;
}
```
在该代码中,我们定义了一个栈的结构体,并实现了栈的初始化、判空、判满、入栈、出栈和查看栈顶元素等基本操作。然后我们定义了两个栈,分别用于存储数字和运算符。在处理表达式时,我们遍历表达式中的每个字符,如果是数字则将其压入数字栈中,如果是运算符则根据其优先级决定是否需要先计算其他运算符,并将该运算符压入运算符栈中。最后,当整个表达式处理完毕后,我们依次从运算符栈中取出运算符,并从数字栈中取出两个数字进行运算,将运算结果压入数字栈中,直到运算符栈为空。最终,数字栈中的唯一元素即为表达式的计算结果。
阅读全文
相关推荐
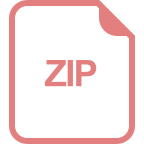
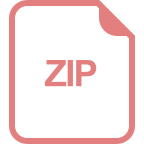















