C# 代码 bgr转为hsi,并标注范围与注释
时间: 2024-10-17 17:07:23 浏览: 34
在C#中,将BGR色彩空间转换成HSI(色相、饱和度、明度)色彩空间通常涉及颜色处理库,如AForge.NET。以下是一个简单的示例代码片段:
```csharp
using AForge.Color;
using System.Drawing;
public class BgrToHisConverter
{
// HSI范围: 色相0-360度,饱和度0-1,明度0-1
public static void ConvertBgrToHsi(Color bgrColor)
{
// 创建一个Bgr到Rgb的颜色转换器
ColorMatrix cm = new ColorMatrix(
new float[][] {
new float[] { 1, 0, 0, 0, 0 },
new float[] { 0, 1, 0, 0, 0 },
new float[] { 0, 0, 1, 0, 0 },
new float[] { 0, 0, 0, 1, 0 }, // 透明度保持不变
new float[] { 0, 0, 0, 0, 1 } // 新通道的偏移量(这里不需要)
});
// 使用矩阵对BGR进行转换
Color hsiColor = ColorMatrixMultiplication.Apply(bgrColor.ToArgb(), cm);
double hue = (hsiColor.R / 255.0) * 6.0 - 0.5; // 计算色相,范围是-0.5到+0.5,然后映射到0-360度
double saturation = hsiColor.G / 255.0; // 饱和度范围是0-1
double intensity = hsiColor.B / 255.0; // 明亮度范围也是0-1
// 返回转换后的HSI值
Console.WriteLine($"HSV: Hue={hue} Saturation={saturation} Intensity={intensity}");
}
private static Color ColorMatrixMultiplication(Color color, ColorMatrix matrix)
{
int r = (int)(color.R * matrix.Matrix33 + color.G * matrix.Matrix34 + color.B * matrix.Matrix35);
int g = (int)(color.R * matrix.Matrix43 + color.G * matrix.Matrix44 + color.B * matrix.Matrix45);
int b = (int)(color.R * matrix.Matrix53 + color.G * matrix.Matrix54 + color.B * matrix.Matrix55);
return Color.FromArgb(color.A, r, g, b);
}
}
// 示例调用
Color bgrColor = Color.Red;
BgrToHisConverter.ConvertBgrToHsi(bgrColor);
```
请注意,这个例子假设输入的是BGR格式的颜色,如果需要RGB格式,只需要改变`ColorMatrix`的构造函数中的系数即可。
阅读全文
相关推荐
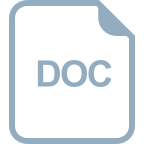
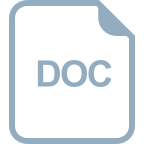
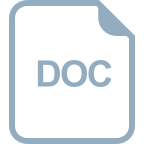

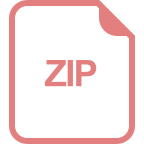
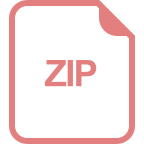
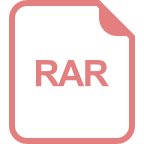
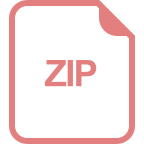
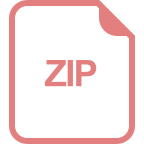
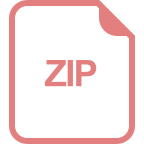








