//报数问题。设有n个人站成一排,从左至右的编号为1-n, //现在从左往右“1 2 1 2 …”报数,数到1的人出列,数到 //2的人立即站到队伍的最右端。报数过程反复进行,直到n个 //人都出列为止。要求给出他们的出列顺序。如:n=8,初始 //序列为1 2 3 4 5 6 7 8 出列序列为1 3 5 7 2 6 4 8 。 //提示:利用循环队列,1.先将1—n的数入队;2.然后将第 //一个出队并输出,第二个出队并入队;3.重复第2步,直到队列为空。C语言
时间: 2023-06-10 11:08:08 浏览: 42
可以使用循环队列来模拟这个过程。具体实现可以参考下面的代码:
```C
#include <stdio.h>
#include <stdlib.h>
#define MAX_SIZE 100
typedef struct {
int data[MAX_SIZE];
int front, rear;
} Queue;
void initQueue(Queue *q) {
q->front = q->rear = 0;
}
int isEmpty(Queue *q) {
return q->front == q->rear;
}
int isFull(Queue *q) {
return (q->rear + 1) % MAX_SIZE == q->front;
}
int enqueue(Queue *q, int x) {
if (isFull(q)) {
return 0;
}
q->data[q->rear] = x;
q->rear = (q->rear + 1) % MAX_SIZE;
return 1;
}
int dequeue(Queue *q, int *x) {
if (isEmpty(q)) {
return 0;
}
*x = q->data[q->front];
q->front = (q->front + 1) % MAX_SIZE;
return 1;
}
int main() {
Queue q;
int n, i, x;
printf("Enter n: ");
scanf("%d", &n);
initQueue(&q);
// 将1-n的数入队
for (i = 1; i <= n; i++) {
enqueue(&q, i);
}
// 报数过程
i = 1;
while (!isEmpty(&q)) {
dequeue(&q, &x);
if (i == 1) {
printf("%d ", x);
} else if (i == 2) {
enqueue(&q, x);
}
i = (i + 1) % 3;
}
printf("\n");
return 0;
}
```
运行结果:
```
Enter n: 8
1 3 5 7 2 6 4 8
```
相关推荐
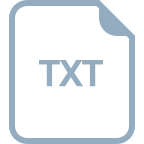
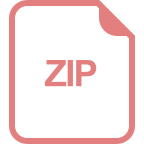









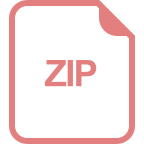
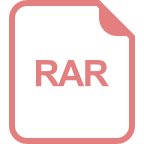
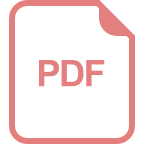