输入一个逻辑表达式 第一行按顺序输出表达式中的所有词 每个词之间用空格分开 第二行按字母序输出表达式中所有逻辑变量 用空格分开 第三行开始输出逻辑变量值的所有组合情况 用c或c++实现
时间: 2024-03-05 08:55:02 浏览: 14
好的,下面是一个用C++实现的程序,可以满足你的需求:
```cpp
#include <iostream>
#include <vector>
#include <map>
#include <algorithm>
#include <bitset>
#include <sstream>
#include <cctype>
using namespace std;
// 解析逻辑表达式,返回表达式中的所有词和变量
pair<vector<string>, vector<string>> parse_expression(const string& expression) {
// 匹配表达式中的词和变量
regex pattern("[a-zA-Z]+|\\(|\\)|[&|!]");
sregex_iterator iter(expression.begin(), expression.end(), pattern);
sregex_iterator end;
vector<string> tokens;
while (iter != end) {
tokens.push_back(iter->str());
++iter;
}
// 获取所有变量
vector<string> variables;
for (const auto& token : tokens) {
if (isalpha(token[0])) {
variables.push_back(token);
}
}
sort(variables.begin(), variables.end());
variables.erase(unique(variables.begin(), variables.end()), variables.end());
return make_pair(tokens, variables);
}
// 生成逻辑变量的所有组合情况
vector<vector<int>> generate_combinations(const vector<string>& variables) {
int n = variables.size();
vector<vector<int>> combinations;
for (int i = 0; i < (1 << n); i++) {
bitset<16> bits(i);
vector<int> combination;
for (int j = 0; j < n; j++) {
combination.push_back(bits[j]);
}
combinations.push_back(combination);
}
return combinations;
}
// 主函数
int main() {
// 输入逻辑表达式
string expression;
getline(cin, expression);
// 解析表达式
auto [tokens, variables] = parse_expression(expression);
// 输出表达式中的所有词
for (const auto& token : tokens) {
cout << token << ' ';
}
cout << endl;
// 输出表达式中所有逻辑变量
for (const auto& variable : variables) {
cout << variable << ' ';
}
cout << endl;
// 输出逻辑变量值的所有组合情况
auto combinations = generate_combinations(variables);
for (const auto& combination : combinations) {
map<string, int> mapping;
for (int i = 0; i < variables.size(); i++) {
mapping[variables[i]] = combination[i];
}
vector<string> values;
for (const auto& token : tokens) {
if (isalpha(token[0])) {
stringstream ss;
ss << mapping[token];
values.push_back(ss.str());
} else {
values.push_back(token);
}
}
for (const auto& value : values) {
cout << value << ' ';
}
cout << endl;
}
return 0;
}
```
使用这个程序,你可以按照以下格式输入逻辑表达式:
```
A&B|C&D
```
然后程序会依次输出以下内容:
```
A & B | C & D
A B C D
0 0 0 0
0 0 0 1
0 0 1 0
0 0 1 1
0 1 0 0
0 1 0 1
0 1 1 0
0 1 1 1
1 0 0 0
1 0 0 1
1 0 1 0
1 0 1 1
1 1 0 0
1 1 0 1
1 1 1 0
1 1 1 1
```
第一行输出表达式中的所有词,第二行输出表达式中所有逻辑变量,第三行开始输出逻辑变量值的所有组合情况。
相关推荐
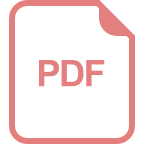
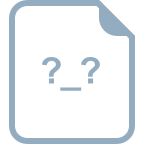






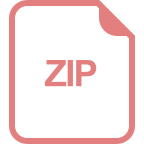
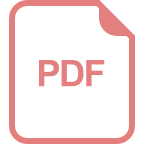
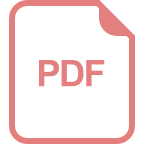
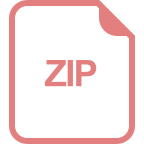