std thread 的 用法举例
时间: 2023-04-08 19:04:19 浏览: 117
std thread 是 C++11 标准库中的一个线程库,可以用于创建和管理线程。下面是一个简单的示例:
```cpp
#include <iostream>
#include <thread>
void printHello() {
std::cout << "Hello from thread!" << std::endl;
}
int main() {
// 创建一个新线程,并执行 printHello 函数
std::thread t(printHello);
// 等待线程执行完毕
t.join();
std::cout << "Hello from main thread!" << std::endl;
return 0;
}
```
在上面的示例中,我们创建了一个新线程,并让它执行 printHello 函数。然后,我们等待线程执行完毕,再输出一条消息。注意,我们必须调用 t.join() 来等待线程执行完毕,否则程序会立即退出,而新线程可能还没有执行完毕。
相关问题
c++ 条件变量的用法举例 ,使用Std:thread
条件变量是一种多线程编程中的同步机制,可以让线程等待某个条件的满足而不需要一直占用CPU资源。条件变量通常是与互斥锁配合使用,以保证线程安全。
以下是使用条件变量和std::thread的例子:
```C++
#include <iostream>
#include <thread>
#include <mutex>
#include <condition_variable>
std::mutex mtx;
std::condition_variable cv;
bool flag = false;
void worker_thread() {
std::unique_lock<std::mutex> lck(mtx);
cv.wait(lck, []{return flag;}); // 等待flag变为true
std::cout << "Worker thread is working" << std::endl;
}
int main() {
std::thread t(worker_thread);
// 延迟一会儿,然后改变flag的值
std::this_thread::sleep_for(std::chrono::seconds(2));
{
std::lock_guard<std::mutex> lck(mtx);
flag = true;
}
cv.notify_one(); // 唤醒等待线程
t.join();
return 0;
}
```
上述代码中,线程t在等待flag变为true时调用了条件变量的wait()方法。当flag变为true时,主线程通过调用条件变量的notify_one()方法唤醒了线程t,线程t继续执行。
注意,在使用条件变量时需要使用std::unique_lock而不是std::lock_guard来构造锁,因为wait()方法需要在持有锁的情况下进行调用。同时,wait()方法应该在while循环内使用,以避免错误地唤醒线程。
c++ 互斥锁用法举例,使用std::thread
A:下面是一个使用std::thread和互斥锁的简单例子:
```cpp
#include <thread>
#include <mutex>
#include <iostream>
std::mutex mtx;
void print(int num) {
mtx.lock(); // 获取互斥锁
std::cout << "Thread " << num << " is printing." << std::endl;
mtx.unlock(); // 释放互斥锁
}
int main() {
std::thread threads[5]; // 创建5个线程
// 创建线程并启动
for (int i = 0; i < 5; ++i) {
threads[i] = std::thread(print, i + 1);
}
// 等待所有线程执行完毕
for (int i = 0; i < 5; ++i) {
threads[i].join();
}
return 0;
}
```
在上述例子中,我们创建了5个线程,并在每个线程中调用print函数。由于print函数里面的输出语句是共享资源,因此需要用互斥锁来保证每个线程访问共享资源的时候都是独占的,避免出现线程安全问题。所以我们在print函数中使用了mtx.lock()来获取互斥锁,输出完毕后再使用mtx.unlock()来释放互斥锁,确保每个线程都可以按照顺序完成自己的任务,不会发生互相干扰的情况。
阅读全文
相关推荐




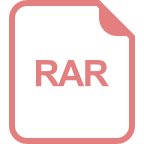









