定义一个矩阵类Matrix,均为M行N列,通过重载运算符“+”、“-”、“<<”、“>>”、“++”、“--”、“==”、“!=”来实现矩阵的相加、相减、输出、输入、自增、自减以及相等、不等的判断。
时间: 2024-11-30 19:12:46 浏览: 33
定义一个矩阵类(Matrix)通常包含以下步骤:
1. 定义类`Matrix`,包含成员变量表示矩阵的行数(rows)、列数(cols)以及一个二维数组存储矩阵元素。
```cpp
class Matrix {
public:
int rows, cols;
std::vector<std::vector<int>> data;
// 构造函数用于初始化矩阵
Matrix(int m, int n) : rows(m), cols(n), data(m, std::vector<int>(n)) {}
};
```
2. 为运算符重载提供实现:
- `+` (相加)
```cpp
Matrix operator+(const Matrix& other) const {
if (rows != other.rows || cols != other.cols) {
throw std::invalid_argument("Matrices must have the same dimensions for addition.");
}
Matrix result(rows, cols);
for (int i = 0; i < rows; ++i) {
for (int j = 0; j < cols; ++j) {
result.data[i][j] = data[i][j] + other.data[i][j];
}
}
return result;
}
```
- `-` (相减)
类似于`+`,只需更改操作符号即可。
- `<<` (输出)
```cpp
friend std::ostream& operator<<(std::ostream& os, const Matrix& matrix) {
for (int i = 0; i < matrix.rows; ++i) {
for (int j = 0; j < matrix.cols; ++j) {
os << matrix.data[i][j] << " ";
}
os << '\n';
}
return os;
}
```
- `>>` (输入)
需要一个接受输入流并更新矩阵数据的方法,这可能涉及到一行一行读取数据,具体取决于如何解析输入。
- `++` 和 `--` (自增/自减)
这些可以通过修改矩阵中的每个元素来实现,但需要注意边界检查和值的变化范围。
- `==` 和 `!=` (相等和不等)
```cpp
bool operator==(const Matrix& other) const {
if (rows != other.rows || cols != other.cols) {
return false;
}
for (int i = 0; i < rows; ++i) {
for (int j = 0; j < cols; ++j) {
if (data[i][j] != other.data[i][j]) {
return false;
}
}
}
return true;
}
bool operator!=(const Matrix& other) const {
return !(*this == other);
}
```
3. 注意,在实际编程中,还需要考虑异常处理,例如当试图对不同维度的矩阵进行操作时。
**相关问题:**
1. 如何处理不同维度的矩阵相加?
2. 输入矩阵时如何确保正确解析?
3. 自增和自减操作如何避免越界或值溢出?
4. 矩阵类的其他友元运算符是否也需要实现?
阅读全文
相关推荐
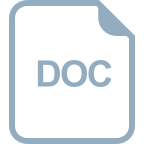
















