设计一个2行3列的矩阵类,重载运算符"+"和"-",能实现矩阵类对象的加减运算;重载流插入运算符"<<“和流提取运算符”>>",使之能用于矩阵的输入和输出。
时间: 2023-07-19 18:22:59 浏览: 224
好的,下面是一个简单的2行3列矩阵类的实现代码,包含了重载运算符和流插入/提取运算符:
```c++
#include <iostream>
using namespace std;
class Matrix {
public:
Matrix() {} // 默认构造函数
Matrix(int m, int n) { // 构造函数
rows = m;
cols = n;
data = new int *[rows];
for (int i = 0; i < rows; i++) {
data[i] = new int[cols];
}
}
~Matrix() { // 析构函数
for (int i = 0; i < rows; i++) {
delete[] data[i];
}
delete[] data;
}
// 重载运算符
Matrix operator+(const Matrix& other) const {
Matrix res(rows, cols);
for (int i = 0; i < rows; i++) {
for (int j = 0; j < cols; j++) {
res.data[i][j] = data[i][j] + other.data[i][j];
}
}
return res;
}
Matrix operator-(const Matrix& other) const {
Matrix res(rows, cols);
for (int i = 0; i < rows; i++) {
for (int j = 0; j < cols; j++) {
res.data[i][j] = data[i][j] - other.data[i][j];
}
}
return res;
}
// 流插入/提取运算符
friend ostream& operator<<(ostream& os, const Matrix& mat) {
for (int i = 0; i < mat.rows; i++) {
for (int j = 0; j < mat.cols; j++) {
os << mat.data[i][j] << " ";
}
os << endl;
}
return os;
}
friend istream& operator>>(istream& is, Matrix& mat) {
for (int i = 0; i < mat.rows; i++) {
for (int j = 0; j < mat.cols; j++) {
is >> mat.data[i][j];
}
}
return is;
}
private:
int rows, cols;
int **data;
};
int main() {
Matrix mat1(2, 3), mat2(2, 3);
// 输入矩阵 mat1 和 mat2
cout << "Please input matrix mat1:" << endl;
cin >> mat1;
cout << "Please input matrix mat2:" << endl;
cin >> mat2;
// 输出矩阵 mat1 和 mat2
cout << "mat1:" << endl;
cout << mat1;
cout << "mat2:" << endl;
cout << mat2;
// 矩阵加减
cout << "mat1 + mat2:" << endl;
cout << mat1 + mat2;
cout << "mat1 - mat2:" << endl;
cout << mat1 - mat2;
return 0;
}
```
这段代码定义了一个 Matrix 类,包含了矩阵的行数和列数,以及矩阵元素的二维数组 data。重载了加减运算符以实现矩阵的加减运算,重载了流插入/提取运算符以实现矩阵的输入和输出。在 main 函数中,我们先输入两个矩阵 mat1 和 mat2,然后输出它们的和、差。
阅读全文
相关推荐
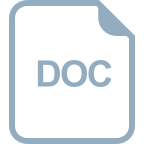
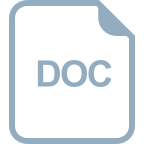
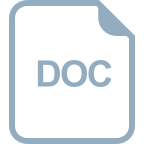








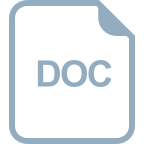






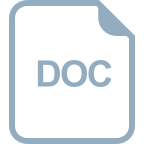