词云expected string or bytes-like object
时间: 2024-06-13 12:06:33 浏览: 208
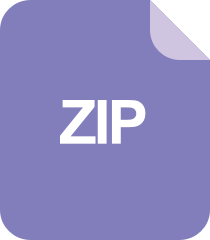
selenium Page Object设计模式与实战
这个错误通常出现在使用词云生成器时,因为它需要一个字符串或类似字节的对象作为输入。如果你遇到了这个错误,可能是因为你的输入不是一个字符串或字节对象。你可以尝试将输入转换为字符串或字节对象,或者检查你的输入是否正确。
以下是一些可能有用的解决方法:
1.确保你的输入是一个字符串或字节对象。如果你的输入是一个列表或其他类型的对象,请将其转换为字符串或字节对象。
2.检查你的输入是否正确。如果你的输入不是一个字符串或字节对象,那么你可能需要重新检查你的代码,以确保你的输入正确。
3.尝试使用Python的内置str()函数将输入转换为字符串。例如,如果你的输入是一个整数,你可以使用str()函数将其转换为字符串。
```python
num = 123
string_num = str(num)
```
阅读全文
相关推荐
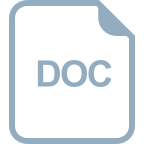
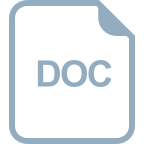



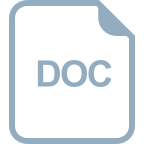
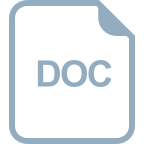
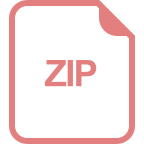
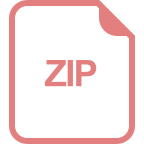
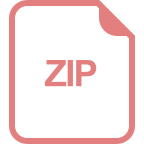
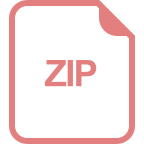
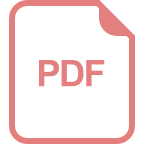
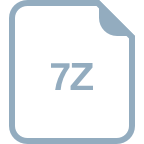
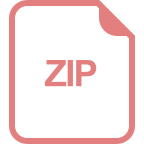
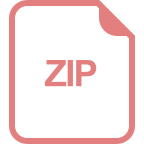
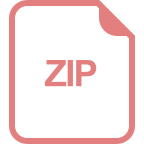
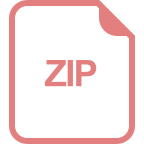
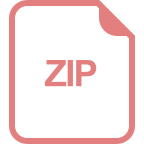