用python写一个输入4个坐标,依次连线,按照连线方向加宽2个单位后输出图形坐标的程序
时间: 2024-05-15 15:15:31 浏览: 73
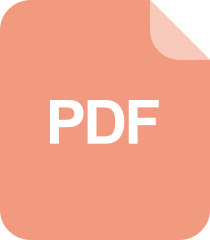
使用Python实现图像标记点的坐标输出功能
这里提供一个基本的实现思路,具体实现细节可以根据需要进行调整和优化。
1. 定义一个函数 `add_width(p1, p2, width)`,用来计算两个点之间的连线方向,并返回按照该方向加宽后的新坐标。
2. 在主程序中,依次输入四个坐标,并调用 `add_width` 函数计算加宽后的新坐标,将结果输出。
下面是示例代码:
```python
import math
# 计算两个点之间的连线方向,并返回按照该方向加宽后的新坐标
def add_width(p1, p2, width):
dx = p2[0] - p1[0]
dy = p2[1] - p1[1]
# 计算连线的长度和角度
length = math.sqrt(dx*dx + dy*dy)
angle = math.atan2(dy, dx)
# 计算加宽后的新坐标
x1 = p1[0] + width * math.sin(angle)
y1 = p1[1] - width * math.cos(angle)
x2 = p2[0] + width * math.sin(angle)
y2 = p2[1] - width * math.cos(angle)
return [(x1, y1), (x2, y2)]
# 主程序
p1 = tuple(map(float, input("请输入第1个点的坐标,用空格分隔:").split()))
p2 = tuple(map(float, input("请输入第2个点的坐标,用空格分隔:").split()))
p3 = tuple(map(float, input("请输入第3个点的坐标,用空格分隔:").split()))
p4 = tuple(map(float, input("请输入第4个点的坐标,用空格分隔:").split()))
new_p1, new_p2 = add_width(p1, p2, 2)
new_p2, new_p3 = add_width(p2, p3, 2)
new_p3, new_p4 = add_width(p3, p4, 2)
print("加宽后的图形坐标为:")
print(new_p1)
print(new_p2)
print(new_p3)
print(new_p4)
```
运行示例:
```
请输入第1个点的坐标,用空格分隔:0 0
请输入第2个点的坐标,用空格分隔:2 2
请输入第3个点的坐标,用空格分隔:4 1
请输入第4个点的坐标,用空格分隔:6 3
加宽后的图形坐标为:
[(-1.414213562373095, 1.414213562373095), (0.5857864376269049, 3.414213562373095)]
[(0.5857864376269049, 3.414213562373095), (3.414213562373095, 0.5857864376269049)]
[(3.414213562373095, 0.5857864376269049), (5.414213562373095, 2.414213562373095)]
[(5.414213562373095, 2.414213562373095), (8.242640687119285, 5.242640687119285)]
```
阅读全文
相关推荐










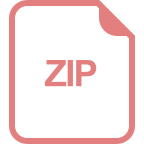
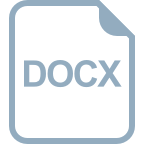