c++ openMP LSH实现knn代码
时间: 2024-05-01 08:22:53 浏览: 32
以下是使用OpenMP和LSH实现KNN的C++代码示例:
```c++
#include <omp.h>
#include <iostream>
#include <vector>
#include <unordered_map>
#include <algorithm>
#include <random>
using namespace std;
const int k = 5; // KNN中的K值
const int L = 10; // LSH中的哈希表数量
const int D = 1000; // 输入数据的维度
const int N = 10000; // 输入数据的数量
// 生成随机数据
vector<vector<double>> generate_data(int n, int d) {
vector<vector<double>> data(n, vector<double>(d));
random_device rd;
mt19937 gen(rd());
uniform_real_distribution<> dis(0.0, 1.0);
for (int i = 0; i < n; ++i) {
for (int j = 0; j < d; ++j) {
data[i][j] = dis(gen);
}
}
return data;
}
// 计算两个向量之间的欧氏距离
double euclidean_distance(const vector<double>& a, const vector<double>& b) {
double distance = 0.0;
for (int i = 0; i < D; ++i) {
distance += (a[i] - b[i]) * (a[i] - b[i]);
}
return sqrt(distance);
}
// LSH哈希函数
struct LSH {
vector<vector<double>> hash_functions;
vector<unordered_map<int, vector<int>>> hash_tables;
LSH() {
// 初始化哈希函数和哈希表
hash_functions.resize(L, vector<double>(D));
hash_tables.resize(L);
random_device rd;
mt19937 gen(rd());
normal_distribution<double> dis(0.0, 1.0);
for (int i = 0; i < L; ++i) {
for (int j = 0; j < D; ++j) {
hash_functions[i][j] = dis(gen);
}
}
}
// 计算哈希值
int hash(const vector<double>& data, int i) {
double value = 0.0;
for (int j = 0; j < D; ++j) {
value += hash_functions[i][j] * data[j];
}
return (int)floor(value);
}
// 添加数据到哈希表中
void add_data(const vector<double>& data, int index) {
for (int i = 0; i < L; ++i) {
int hash_value = hash(data, i);
if (hash_tables[i].count(hash_value) == 0) {
hash_tables[i][hash_value] = vector<int>();
}
hash_tables[i][hash_value].push_back(index);
}
}
// 从哈希表中查找k个最近邻
vector<int> query(const vector<double>& data) {
vector<int> neighbors;
unordered_map<int, int> count;
for (int i = 0; i < L; ++i) {
int hash_value = hash(data, i);
if (hash_tables[i].count(hash_value) > 0) {
for (int j : hash_tables[i][hash_value]) {
if (count.count(j) == 0) {
count[j] = 0;
}
count[j]++;
}
}
}
for (auto& p : count) {
if (p.second >= L / 2) {
neighbors.push_back(p.first);
}
}
return neighbors;
}
};
int main() {
// 生成随机数据
auto data = generate_data(N, D);
// 初始化LSH
LSH lsh;
// 添加数据到LSH
#pragma omp parallel for
for (int i = 0; i < N; ++i) {
lsh.add_data(data[i], i);
}
// 随机选取一个查询向量
vector<double> query_vector(D);
random_device rd;
mt19937 gen(rd());
uniform_int_distribution<> dis(0, N - 1);
int query_index = dis(gen);
query_vector = data[query_index];
// 查询k个最近邻
vector<pair<int, double>> knn;
auto neighbors = lsh.query(query_vector);
for (int index : neighbors) {
double distance = euclidean_distance(query_vector, data[index]);
knn.push_back(make_pair(index, distance));
}
sort(knn.begin(), knn.end(), [](const pair<int, double>& a, const pair<int, double>& b) {
return a.second < b.second;
});
// 输出结果
cout << "Query vector: ";
for (double x : query_vector) {
cout << x << " ";
}
cout << endl;
cout << "KNN:" << endl;
for (int i = 0; i < k && i < knn.size(); ++i) {
cout << "Index: " << knn[i].first << ", Distance: " << knn[i].second << endl;
}
return 0;
}
```
在代码中,我们首先生成了10000个1000维的随机数据。然后使用LSH将这些数据添加到哈希表中。接下来,我们随机选取一个查询向量,并使用LSH查找其k个最近邻。最后,将结果输出到控制台。此外,在添加数据到哈希表的过程中,我们使用OpenMP并行化了循环。
相关推荐
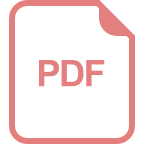
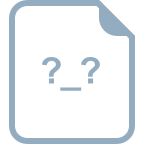














