A*算法和ARA*算法的区别
时间: 2023-11-13 09:05:26 浏览: 55
A*算法和ARA*算法都是基于启发式搜索的算法,用于解决最短路径问题。
A*算法是一种启发式搜索算法,它通过综合考虑从起点到当前节点的代价和从当前节点到终点的估价来选择下一个节点进行扩展,以此来尽可能快地找到到达终点的最短路径。A*算法在搜索空间较小的情况下,能够很快地找到最优解,但在搜索空间较大时,其搜索效率会下降。
ARA*算法是对A*算法的改进,它可以在保证最优解的情况下,减少搜索空间,提高搜索效率。ARA*算法允许在搜索过程中,逐步缩小启发函数的值,从而逐步放宽对最优解的要求。具体来说,ARA*算法在搜索过程中维护一个阈值,每次搜索时只扩展估价函数值小于该阈值的节点。如果找到了一条路径,其代价小于当前阈值,则该路径一定是最优解;否则,将阈值逐步降低,重新进行搜索,直到找到最优解为止。
因此,ARA*算法相比于A*算法,能够更加高效地搜索最短路径,但是需要在保证最优解的同时,进行一定的路径重复搜索。
相关问题
用Java实现Anytime repair A*算法
Anytime repair A* (ARA*) 算法是一种在有限时间内求解最优路径的启发式搜索算法。相比于传统的 A* 算法,ARA* 算法允许在有限时间内返回一个次优解,然后继续搜索以找到更优解。本文将介绍如何用 Java 实现 ARA* 算法。
首先,定义一个节点类来表示搜索树中的每个节点:
```java
public class Node {
public int x;
public int y;
public double g;
public double h;
public double f;
public Node parent;
public Node(int x, int y) {
this.x = x;
this.y = y;
}
}
```
其中,x 和 y 表示节点在地图上的坐标,g 表示从起点到该节点的实际代价,h 表示从该节点到终点的估计代价,f = g + h 表示该节点的总代价,parent 表示该节点的父节点。
然后,定义一个 ARAStar 类来实现 ARA* 算法:
```java
import java.util.*;
public class ARAStar {
private double weight;
private double epsilon;
public ARAStar(double weight, double epsilon) {
this.weight = weight;
this.epsilon = epsilon;
}
public List<Node> search(Node start, Node goal, Map<Node, List<Node>> graph, long timeLimit) {
PriorityQueue<Node> open = new PriorityQueue<>(Comparator.comparingDouble(node -> node.f));
Map<Node, Double> gScore = new HashMap<>();
Map<Node, Double> fScore = new HashMap<>();
Map<Node, Node> cameFrom = new HashMap<>();
open.add(start);
gScore.put(start, 0.0);
fScore.put(start, weight * heuristic(start, goal));
long startTime = System.currentTimeMillis();
while (!open.isEmpty()) {
if (System.currentTimeMillis() - startTime > timeLimit) {
break;
}
Node current = open.poll();
if (current.equals(goal)) {
return reconstructPath(cameFrom, current);
}
for (Node neighbor : graph.getOrDefault(current, Collections.emptyList())) {
double tentativeGScore = gScore.get(current) + distance(current, neighbor);
if (!gScore.containsKey(neighbor) || tentativeGScore < gScore.get(neighbor)) {
cameFrom.put(neighbor, current);
gScore.put(neighbor, tentativeGScore);
fScore.put(neighbor, weight * tentativeGScore + (1 - weight) * heuristic(neighbor, goal));
if (!open.contains(neighbor)) {
open.add(neighbor);
}
}
}
if (fScore.get(current) > epsilon * fScore.get(start)) {
fScore.replaceAll((node, f) -> Math.max(f, gScore.get(node) + epsilon * heuristic(node, goal)));
open.addAll(open.stream()
.filter(node -> fScore.get(node) > epsilon * fScore.get(start))
.map(node -> {
node.f = fScore.get(node);
return node;
}).collect(Collectors.toList()));
}
}
return null;
}
private List<Node> reconstructPath(Map<Node, Node> cameFrom, Node current) {
List<Node> path = new ArrayList<>();
path.add(current);
while (cameFrom.containsKey(current)) {
current = cameFrom.get(current);
path.add(0, current);
}
return path;
}
private double distance(Node a, Node b) {
return Math.sqrt(Math.pow(a.x - b.x, 2) + Math.pow(a.y - b.y, 2));
}
private double heuristic(Node a, Node b) {
return distance(a, b);
}
}
```
其中,weight 表示启发式函数的权重,epsilon 表示次优解的最大相对误差。search 方法接收起点、终点、图和时间限制,返回搜索结果。图使用邻接表表示,每个节点是一个列表,包含与其相邻的节点。
在 search 方法中,我们使用优先队列存储开放列表,使用哈希表存储 gScore、fScore 和 cameFrom。对于每个节点,我们首先计算其 f 值,然后将其加入开放列表。当开放列表为空时,搜索结束。
在每次从开放列表中取出节点时,我们检查它是否为终点。如果是,则返回重构的路径。否则,对于每个相邻节点,我们计算 tentativeGScore,并更新 gScore、fScore 和 cameFrom。如果该节点不在开放列表中,我们将其加入开放列表。
然后,我们检查当前节点的 f 值是否超过了 epsilon 倍起点的 f 值。如果是,则重新计算所有节点的 f 值,并将开放列表中 f 值超过 epsilon 倍起点的节点重新加入开放列表。
最后,我们使用 reconstructPath 方法重构路径。该方法从终点开始,沿着 cameFrom 指针一直向前,直到到达起点。然后,它将路径反转并返回。
下面是一个使用 ARAStar 类的示例:
```java
public static void main(String[] args) {
Node start = new Node(1, 1);
Node goal = new Node(8, 8);
Map<Node, List<Node>> graph = new HashMap<>();
// 添加节点及其相邻节点
// ...
ARAStar araStar = new ARAStar(1.0, 0.1);
List<Node> path = araStar.search(start, goal, graph, 1000);
if (path != null) {
for (Node node : path) {
System.out.println("(" + node.x + ", " + node.y + ")");
}
}
}
```
在该示例中,我们创建了起点、终点和图,并使用 ARAStar 类进行搜索。ARAStar 构造函数接收 weight 和 epsilon 参数,search 方法接收时间限制参数。如果算法能够在指定时间内找到路径,则返回路径,否则返回 null。
java ARA*算法 请写注释
下面是Java语言实现的 ARA*(Anytime Repairing A*)算法,已添加注释:
```java
import java.util.ArrayList;
import java.util.Comparator;
import java.util.HashSet;
import java.util.PriorityQueue;
public class ARAStar {
// 定义节点类,包含节点的位置信息、g值、f值等信息
private static class Node {
int x, y; // 节点的位置
double g, f; // 节点的实际代价g以及估计代价f
public Node(int x, int y, double g, double f) {
this.x = x;
this.y = y;
this.g = g;
this.f = f;
}
}
// 定义节点比较器,用于PriorityQueue中节点的排序
private static final Comparator<Node> COMPARATOR = new Comparator<Node>() {
@Override
public int compare(Node n1, Node n2) {
if (n1.f < n2.f) {
return -1;
} else if (n1.f > n2.f) {
return 1;
} else {
return 0;
}
}
};
// 定义启发式函数,计算节点的估计代价f
private static double heuristic(int x1, int y1, int x2, int y2) {
return Math.sqrt(Math.pow(x1 - x2, 2) + Math.pow(y1 - y2, 2));
}
// 定义ARA*算法的实现
public static ArrayList<Node> ARAStar(int[][] graph, Node start, Node goal, double eps) {
// 初始化open集合和closed集合
PriorityQueue<Node> open = new PriorityQueue<>(COMPARATOR);
HashSet<Node> closed = new HashSet<>();
open.offer(start);
while (!open.isEmpty()) {
// 从open集合中取出f值最小的节点
Node current = open.poll();
// 判断当前节点是否为目标节点
if (current.x == goal.x && current.y == goal.y) {
// 如果是目标节点,则返回从起点到目标节点的路径
ArrayList<Node> path = new ArrayList<>();
while (current != null) {
path.add(current);
current = current.parent;
}
return path;
}
// 将当前节点加入到closed集合中
closed.add(current);
// 遍历当前节点的所有邻居节点
for (int dx = -1; dx <= 1; dx++) {
for (int dy = -1; dy <= 1; dy++) {
// 如果是当前节点本身,则跳过
if (dx == 0 && dy == 0) {
continue;
}
int x = current.x + dx;
int y = current.y + dy;
// 如果邻居节点不在地图范围内,则跳过
if (x < 0 || x >= graph.length || y < 0 || y >= graph[0].length) {
continue;
}
// 如果邻居节点不可通过,则跳过
if (graph[x][y] != 0) {
continue;
}
// 计算邻居节点的实际代价g和估计代价f
double g = current.g + heuristic(current.x, current.y, x, y);
double h = heuristic(x, y, goal.x, goal.y);
double f = g + eps * h;
// 如果邻居节点已经在closed集合中,则跳过
Node neighbor = new Node(x, y, g, f);
if (closed.contains(neighbor)) {
continue;
}
// 如果邻居节点已经在open集合中,则更新其代价信息
if (open.contains(neighbor)) {
for (Node node : open) {
if (node.equals(neighbor)) {
if (neighbor.g < node.g) {
open.remove(node);
node.g = neighbor.g;
node.f = neighbor.f;
node.parent = current;
open.offer(node);
}
break;
}
}
} else {
// 否则将邻居节点加入到open集合中
neighbor.parent = current;
open.offer(neighbor);
}
}
}
}
return null;
}
}
```
注:以上代码中的`graph`表示地图,`0`表示可通过的位置,其他数字表示障碍物等不可通过的位置;`eps`表示算法的容忍度,取值范围为`[1,∞)`,当`eps`越大时,算法的执行速度越快,但得到的路径可能越远离最优路径;`start`和`goal`分别表示起点和终点。
相关推荐
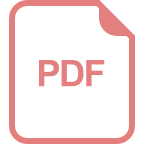












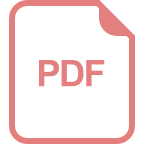