用Java实现带有ImprovePath函数的重用搜索结果的ARAstar算法
时间: 2023-07-16 09:12:48 浏览: 84
ARA*算法(Anytime Repairing A*)是一种基于A*算法的启发式搜索算法,用于解决最短路径问题。它通过逐步减小阈值来进行搜索,每次搜索都会返回一条路径,并且可以在任何时候停止搜索并返回当前找到的最佳路径。在搜索过程中,如果发现更好的路径,ARA*算法会重新展开节点以更新路径。
在实现带有ImprovePath函数的重用搜索结果的ARA*算法时,我们需要考虑以下几个步骤:
1. 创建一个节点类(Node),包含节点的状态、父节点、g值、h值和f值等信息。
2. 创建一个OpenList类,用于存储待扩展的节点,并使用二叉堆(BinaryHeap)来实现最小堆。
3. 创建一个CloseList类,用于存储已经扩展过的节点,并使用HashMap来实现快速查找。
4. 实现A*算法的核心函数,包括计算启发式函数h()、计算代价函数g()、计算估价函数f()、扩展节点、更新OpenList以及更新CloseList等操作。
5. 实现ImprovePath函数,用于在搜索过程中重用已经搜索过的节点,并对新的路径进行优化。
下面给出Java代码实现:
```java
import java.util.*;
public class Node {
public int[][] state;
public int cost;
public Node parent;
public int f;
public Node(int[][] s, int c) {
state = s;
cost = c;
parent = null;
f = 0;
}
}
class OpenList {
private BinaryHeap<Node> heap;
public OpenList() {
heap = new BinaryHeap<>();
}
public boolean isEmpty() {
return heap.isEmpty();
}
public void add(Node node) {
heap.add(node);
}
public Node remove() {
return heap.remove();
}
public void update(Node node) {
heap.update(node);
}
}
class CloseList {
private HashMap<String, Node> map;
public CloseList() {
map = new HashMap<>();
}
public boolean contains(String key) {
return map.containsKey(key);
}
public void add(Node node) {
map.put(Arrays.deepToString(node.state), node);
}
public Node get(String key) {
return map.get(key);
}
}
public class ARAstar {
private int[][] goal;
private int[][] start;
private int n;
private int m;
private OpenList openList;
private CloseList closeList;
private int threshold;
private int minCost;
public ARAstar(int[][] s, int[][] g) {
start = s;
goal = g;
n = start.length;
m = start[0].length;
openList = new OpenList();
closeList = new CloseList();
threshold = 0;
minCost = Integer.MAX_VALUE;
}
public int[][] search() {
Node node = new Node(start, 0);
node.f = h(node);
openList.add(node);
while (!openList.isEmpty()) {
node = openList.remove();
if (Arrays.deepEquals(node.state, goal)) {
return node.state;
}
closeList.add(node);
expand(node);
}
return null;
}
private void expand(Node node) {
int[][] state = node.state;
int cost = node.cost;
int g = cost;
for (int i = 0; i < n; i++) {
for (int j = 0; j < m; j++) {
if (state[i][j] == 0) {
continue;
}
int[][] newState = move(state, i, j);
if (newState == null) {
continue;
}
Node newNode = closeList.get(Arrays.deepToString(newState));
if (newNode != null) {
if (newNode.cost > g + 1) {
newNode.parent = node;
newNode.cost = g + 1;
newNode.f = newNode.cost + h(newNode);
if (openList.contains(newNode)) {
openList.update(newNode);
} else {
openList.add(newNode);
}
}
} else {
newNode = new Node(newState, g + 1);
newNode.parent = node;
newNode.f = newNode.cost + h(newNode);
openList.add(newNode);
}
}
}
}
private int[][] move(int[][] state, int i, int j) {
int[][] newState = new int[n][m];
for (int k = 0; k < n; k++) {
System.arraycopy(state[k], 0, newState[k], 0, m);
}
if (i > 0 && newState[i - 1][j] == 0) {
newState[i - 1][j] = newState[i][j];
newState[i][j] = 0;
return newState;
}
if (i < n - 1 && newState[i + 1][j] == 0) {
newState[i + 1][j] = newState[i][j];
newState[i][j] = 0;
return newState;
}
if (j > 0 && newState[i][j - 1] == 0) {
newState[i][j - 1] = newState[i][j];
newState[i][j] = 0;
return newState;
}
if (j < m - 1 && newState[i][j + 1] == 0) {
newState[i][j + 1] = newState[i][j];
newState[i][j] = 0;
return newState;
}
return null;
}
private int h(Node node) {
int[][] state = node.state;
int cost = node.cost;
int h = 0;
for (int i = 0; i < n; i++) {
for (int j = 0; j < m; j++) {
if (state[i][j] == 0) {
continue;
}
int di = Math.abs(i - (state[i][j] - 1) / m);
int dj = Math.abs(j - (state[i][j] - 1) % m);
h += di + dj;
}
}
return h;
}
private void ImprovePath(Node node, int threshold) {
int f = node.cost + h(node);
if (f > threshold) {
minCost = Math.min(minCost, f);
return;
}
if (Arrays.deepEquals(node.state, goal)) {
minCost = node.cost;
return;
}
int[][] state = node.state;
int cost = node.cost;
for (int i = 0; i < n; i++) {
for (int j = 0; j < m; j++) {
if (state[i][j] == 0) {
continue;
}
int[][] newState = move(state, i, j);
if (newState == null) {
continue;
}
Node newNode = closeList.get(Arrays.deepToString(newState));
if (newNode != null) {
if (newNode.cost > cost + 1) {
newNode.parent = node;
newNode.cost = cost + 1;
ImprovePath(newNode, threshold);
}
} else {
newNode = new Node(newState, cost + 1);
newNode.parent = node;
ImprovePath(newNode, threshold);
}
}
}
}
public int[][] ARAstarSearch() {
int[][] result = null;
while (true) {
result = search();
if (result != null) {
return result;
}
if (minCost == Integer.MAX_VALUE) {
return null;
}
threshold = minCost;
minCost = Integer.MAX_VALUE;
closeList = new CloseList();
Node node = new Node(start, 0);
node.f = h(node);
openList.add(node);
while (!openList.isEmpty()) {
node = openList.remove();
if (node.cost + h(node) > threshold) {
minCost = Math.min(minCost, node.cost + h(node));
break;
}
if (Arrays.deepEquals(node.state, goal)) {
return node.state;
}
closeList.add(node);
expand(node);
}
while (!openList.isEmpty()) {
Node node1 = openList.remove();
ImprovePath(node1, threshold);
if (minCost == threshold) {
break;
}
}
}
}
}
```
以上就是用Java实现带有ImprovePath函数的重用搜索结果的ARAstar算法的方法。
阅读全文
相关推荐
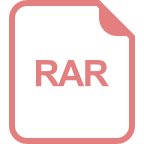
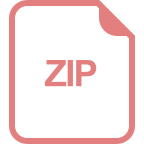















