img = cv2.imdecode(fromfile(img_path, dtype=uint8), cv2.IMREAD_COLOR)
时间: 2024-04-26 21:21:10 浏览: 149
这段代码是使用 OpenCV 库读取指定路径的图像文件,并解码为对应的 NumPy 数组格式。具体来说,cv2.imdecode() 函数接收两个参数:第一个是从文件中读取的二进制数据,第二个是指定解码方式的参数。在这里,我们使用 cv2.IMREAD_COLOR 参数指定解码为彩色图片。解码后的结果存储在 img 变量中,可以通过 NumPy 数组的方式对其进行进一步处理和操作。
相关问题
if img_path: img = cv2.imdecode(fromfile(img_path, dtype=uint8), cv2.IMREAD_COLOR)#彩色 #使用OpenCV库读取指定路径的图像文件,并解码为对应的NumPy数组格式 self.show(img, 400, oriImg) colors, lisencePlates = self.getROI(img) for m in range(len(lisencePlates)): self.show(lisencePlates[m], 40, ROIImg) letters = self.getLetters(lisencePlates[m], colors[m]) results = [] for letter in letters: feature = self.getFeature(letter) result = self.sort(feature, trainingMat, labels, 5) results.append(result) print(result) recogResult = ','.join(results) resultShow.configure(text=recogResult)
这段代码是一个 Python 类中的一个方法,用于处理车牌识别的流程。具体来说,它会读取指定路径的图像文件,解码为对应的 NumPy 数组格式,并进行一系列的处理,最终得到车牌识别的结果。
首先,代码会通过 cv2.imdecode() 函数读取指定路径的图像文件,并解码为彩色图片格式。接着,代码会调用 self.show() 方法,将读取到的图片显示在界面上。然后,代码会调用 self.getROI() 方法,对图片进行车牌的定位和提取,得到一个包含所有车牌的列表 lisencePlates。
接下来,代码会遍历 lisencePlates 中的所有车牌,依次调用 self.show() 方法将车牌显示在界面上,并调用 self.getLetters() 方法提取车牌中的字符。提取过程中,代码会使用 colors 列表记录车牌中每个字符的颜色信息。
对于每个字符,代码会调用 self.getFeature() 方法提取其特征,并调用 self.sort() 方法进行分类。分类过程中,代码会使用训练集的特征矩阵 trainingMat 和标签 labels 进行训练,并选取距离最近的 5 个样本进行分类。分类结果将被记录在 results 列表中。
最后,代码会将 results 中的分类结果拼接成一个字符串 recogResult,并将其显示在界面上。
没有GPU,优化程序class point_cloud_generator(): def init(self, rgb_file, depth_file, save_ply, camera_intrinsics=[312.486, 243.928, 382.363, 382.363]): self.rgb_file = rgb_file self.depth_file = depth_file self.save_ply = save_ply self.rgb = cv2.imread(rgb_file) self.depth = cv2.imread(self.depth_file, -1) print("your depth image shape is:", self.depth.shape) self.width = self.rgb.shape[1] self.height = self.rgb.shape[0] self.camera_intrinsics = camera_intrinsics self.depth_scale = 1000 def compute(self): t1 = time.time() depth = np.asarray(self.depth, dtype=np.uint16).T self.Z = depth / self.depth_scale fx, fy, cx, cy = self.camera_intrinsics X = np.zeros((self.width, self.height)) Y = np.zeros((self.width, self.height)) for i in range(self.width): X[i, :] = np.full(X.shape[1], i) self.X = ((X - cx / 2) * self.Z) / fx for i in range(self.height): Y[:, i] = np.full(Y.shape[0], i) self.Y = ((Y - cy / 2) * self.Z) / fy data_ply = np.zeros((6, self.width * self.height)) data_ply[0] = self.X.T.reshape(-1)[:self.width * self.height] data_ply[1] = -self.Y.T.reshape(-1)[:self.width * self.height] data_ply[2] = -self.Z.T.reshape(-1)[:self.width * self.height] img = np.array(self.rgb, dtype=np.uint8) data_ply[3] = img[:, :, 0:1].reshape(-1)[:self.width * self.height] data_ply[4] = img[:, :, 1:2].reshape(-1)[:self.width * self.height] data_ply[5] = img[:, :, 2:3].reshape(-1)[:self.width * self.height] self.data_ply = data_ply t2 = time.time() print('calcualte 3d point cloud Done.', t2 - t1) def write_ply(self): start = time.time() float_formatter = lambda x: "%.4f" % x points = [] for i in self.data_ply
It that the code is generating a point cloud from an RGB-D image pair. Since you mentioned that you do not have a GPU, one possible optimization could be to use the `numba` library to speed up the computation. Here is how you can modify the code to use `numba`:
1. Import the `numba` library by adding the following line at the top of your code:
```python
import numba
```
2. Add the `@numba.jit(nopython=True)` decorator to the `compute` method to enable `numba` JIT compilation:
```python
@numba.jit(nopython=True)
def compute(self):
# rest of the code
```
3. Modify the `for` loops in the `compute` method to use `numba`'s `prange` function to parallelize the computation across multiple CPU cores:
```python
for i in numba.prange(self.width):
# rest of the code
for i in numba.prange(self.height):
# rest of the code
```
4. Add the `@numba.jit(nopython=True)` decorator to the `write_ply` method as well, since it is also computationally intensive:
```python
@numba.jit(nopython=True)
def write_ply(self):
# rest of the code
```
With these modifications, the `compute` and `write_ply` methods should run faster. However, keep in mind that `numba`'s JIT compilation can take some time during the first function call, so subsequent calls should be faster. Also, note that `numba`'s `prange` function only works with integer indices, so you may need to convert floating-point indices to integers before using them in the loops.
阅读全文
相关推荐
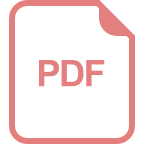
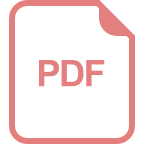
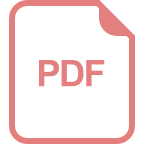













