cv2.imdecode(np.fromfile(filename, dtype=np.uint8), cv2.IMREAD_COLOR
时间: 2024-05-27 11:14:34 浏览: 154
This line of code reads an image file from the file system and decodes it into a NumPy array using OpenCV's `imdecode` function. The `np.fromfile` function reads the contents of the file into a NumPy array of unsigned 8-bit integers (`dtype=np.uint8`). The resulting NumPy array is then passed to the `imdecode` function with the flag `cv2.IMREAD_COLOR` to indicate that the image should be loaded in color mode. The output of this line is a NumPy array representing the decoded image.
相关问题
from skimage.segmentation import slic from skimage.segmentation import mark_boundaries from skimage.util import img_as_float import matplotlib.pyplot as plt import numpy as np import cv2 # args args = {"image": 'I:\\18Breakageratecalculation\\SVM run\\images\\030.jpg'} # load the image and apply SLIC and extract (approximately) # the supplied number of segments image = cv2.imread(args["image"]) segments = slic(img_as_float(image), n_segments=100, sigma=3) # show the output of SLIC fig = plt.figure('Superpixels') ax = fig.add_subplot(1, 1, 1) ax.imshow(mark_boundaries(img_as_float(cv2.cvtColor(image, cv2.COLOR_BGR2RGB)), segments)) plt.axis("off") plt.show() print("segments:\n", segments) print("np.unique(segments):", np.unique(segments)) # loop over the unique segment values for (i, segVal) in enumerate(np.unique(segments)): # construct a mask for the segment print("[x] inspecting segment {}, for {}".format(i, segVal)) mask = np.zeros(image.shape[:2], dtype="uint8") mask[segments == segVal] = 255 # show the masked region cv2.imshow("Mask", mask) cv2.imshow("Applied", np.multiply(image, cv2.cvtColor(mask, cv2.COLOR_GRAY2BGR) > 0)) cv2.waitKey(0) cv2.imwrite("segment_%d.png" % i, np.multiply(image, cv2.cvtColor(mask, cv2.COLOR_GRAY2BGR) > 0))导出子块图像到指定文件夹
可以使用以下代码将每个超像素块保存为单独的图像文件:
```
import os
# create a directory to store the segmented images
if not os.path.exists("segmented_images"):
os.makedirs("segmented_images")
# loop over the unique segment values
for (i, segVal) in enumerate(np.unique(segments)):
# construct a mask for the segment
mask = np.zeros(image.shape[:2], dtype="uint8")
mask[segments == segVal] = 255
# apply the mask to the image
masked_image = np.multiply(image, cv2.cvtColor(mask, cv2.COLOR_GRAY2BGR) > 0)
# save the masked image as a file
filename = os.path.join("segmented_images", "segment_%d.png" % i)
cv2.imwrite(filename, masked_image)
```
这将在当前目录下创建一个名为 "segmented_images" 的文件夹,并将每个超像素块保存为单独的图像文件。
cv2.imdecode 灰度读取
cv2.imdecode函数可以用于将图像数据解码为OpenCV中的图像格式。要以灰度模式读取图像,可以使用以下代码:
```python
import cv2
import numpy as np
# 读取图像数据
image_data = np.fromfile('image.jpg', dtype=np.uint8)
# 解码为灰度图像
gray_image = cv2.imdecode(image_data, cv2.IMREAD_GRAYSCALE)
# 显示灰度图像
cv2.imshow('Gray Image', gray_image)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
在这个例子中,我们首先使用`np.fromfile`函数从文件中读取图像数据,并将其存储在`image_data`变量中。然后,使用`cv2.imdecode`函数解码图像数据为灰度图像,并将结果存储在`gray_image`变量中。最后,使用`cv2.imshow`函数显示灰度图像,并使用`cv2.waitKey`和`cv2.destroyAllWindows`等函数来控制显示窗口的行为。
请注意,此代码假设您已经安装了OpenCV库,并且存在名为'image.jpg'的图像文件。您需要根据实际情况修改文件名和路径。
阅读全文
相关推荐
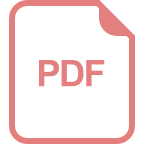
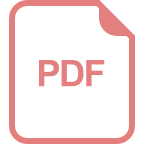














