用python写用最大类间方差法对一张图片进行改变阈值观察图像分割结果变化
时间: 2024-11-17 19:28:22 浏览: 23
在Python中,你可以使用skimage库来进行图像处理,包括阈值处理和类间方差计算。最大类间方差法(Otsu's Method)是一种常用的二值化算法,用于自动确定最佳阈值,将图像分为前景和背景。
首先,你需要安装`skimage`和`matplotlib`这两个库,可以使用pip进行安装:
```bash
pip install scikit-image matplotlib
```
下面是一个简单的例子,展示如何使用Otsu's Method对图片进行阈值调整并观察分割效果的变化:
```python
from skimage import io, filters, exposure
import matplotlib.pyplot as plt
# 加载图片
img = io.imread('your_image_path', as_gray=True)
# Otsu's Method
threshold = filters.threshold_otsu(img)
binary_img = img > threshold
# 显示原图、二值化前的预处理图和二值化后的结果
fig, axs = plt.subplots(1, 3, figsize=(15, 5))
axs[0].imshow(img, cmap='gray')
axs[0].set_title('Original Image')
# 预处理图
preprocessed_img = exposure.rescale_intensity(img, in_range='auto')
axs[1].imshow(preprocessed_img, cmap='gray')
axs[1].set_title('Preprocessed Image (Rescaled Intensity)')
# 二值化后的图像
axs[2].imshow(binary_img, cmap='gray', interpolation='nearest')
axs[2].set_title('Segmented Image (Threshold: {})'.format(threshold))
for ax in axs:
ax.set_axis_off()
plt.show()
# 可以通过修改阈值并再次运行这个过程,观察分割结果的变化
thresholds = [threshold * i for i in range(0, 11)] # 创建一系列阈值
for t in thresholds:
binary_img = img > t
axs[2].clear() # 清除当前轴的内容
axs[2].imshow(binary_img, cmap='gray', interpolation='nearest')
axs[2].set_title('Segmented with Threshold: {}'.format(t))
plt.pause(0.5) # 暂停一段时间以便观察变化
```
在这个示例中,我们首先读取灰度图像,然后应用Otsu's Method获取阈值,并将其应用到原始图像上生成二值图像。接着,我们可以改变阈值并显示不同阈值下的分割结果。
阅读全文
相关推荐
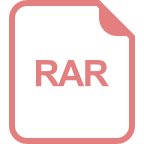
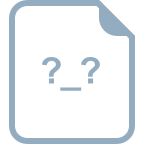









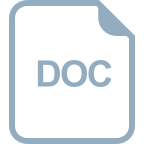



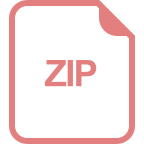

