假定用于通信的电文仅由a,b,c,d,e,f,g,h共8个字符组成。字母在电文中出现的频率分别为: 0.07、0.19、0.02、0.06、0.32、0.03、0.21、0.10。编程构建哈夫曼树,并为这些字母进行编码,并给出代码
时间: 2024-11-20 20:34:35 浏览: 15
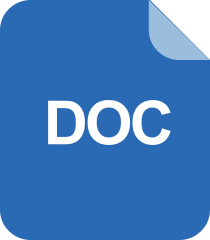
所谓进程,是操作系统中的一个概念
为了构建哈夫曼树并为这些字母编码,我们可以按照以下步骤进行:
1. **计算频率**: 首先,我们需要根据给定的频率创建一个字典,键是字符,值是频率。
```python
frequency = {
'a': 0.07,
'b': 0.19,
'c': 0.02,
'd': 0.06,
'e': 0.32,
'f': 0.03,
'g': 0.21,
'h': 0.10,
}
```
2. **构建优先队列(二叉堆)**: 将字符及其频率放入一个最小堆中,每次取出频率最低的两棵树节点合并成一个新的节点,新的节点的频率是两个子节点的频率之和。
3. **构建哈夫曼树**: 重复上述过程,直到只剩下一个节点,这即为哈夫曼树的根节点。
4. **编码规则**: 从左到右遍历哈夫曼树,遇到分支则向左走代表`0`,向右走代表`1`。将每个字符对应的路径转换为二进制数作为它的编码。
下面是一个简单的Python代码示例,使用了heapq模块来实现最小堆操作:
```python
import heapq
def build_huffman_tree(frequencies):
heap = [[freq, [char, ""]] for char, freq in frequencies.items()]
heapq.heapify(heap)
while len(heap) > 1:
low_freq_left = heapq.heappop(heap)
low_freq_right = heapq.heappop(heap)
combined_freq = low_freq_left[0] + low_freq_right[0]
combined_char = ''.join(low_freq_left[1:] + low_freq_right[1:])
heapq.heappush(heap, [combined_freq, combined_char])
return heap[0][1]
def huffman_encoding(tree, characters):
codes = {}
def traverse(node, code=""):
if isinstance(node, str):
codes[node] = code
else:
traverse(node[0], code + "0")
traverse(node[1], code + "1")
traverse(tree)
return codes
# 使用频率数据构建哈夫曼树
tree = build_huffman_tree(frequency)
codes = huffman_encoding(tree, list(frequency.keys()))
print("哈夫曼树编码:")
for char, code in codes.items():
print(f"{char}: {code}")
```
运行此代码将输出每个字符的哈夫曼编码。请注意,实际的哈夫曼树结构会因随机性而略有不同,因此生成的编码也可能会有所不同。
阅读全文
相关推荐
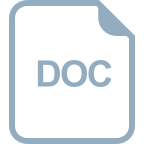
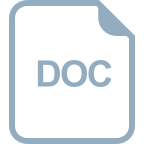















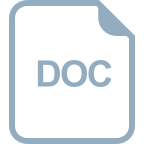