RANSAC拟合二维圆
时间: 2024-12-19 18:06:48 浏览: 6
RANSAC(Random Sample Consensus)是一种迭代算法,用于从包含异常值的数据集中估计数学模型。RANSAC算法通过随机选择数据点的子集来估计模型参数,并评估这些参数在所有数据点上的拟合效果。以下是使用RANSAC拟合二维圆的步骤:
1. **初始化参数**:
- 设置最大迭代次数`N`。
- 设置拟合模型所需的最少数据点数量`n`(对于圆,`n=3`)。
- 设置阈值`t`,用于判断数据点是否在模型内。
- 设置期望的内点数`d`。
2. **迭代过程**:
- 在每次迭代中,随机选择`n`个数据点。
- 使用这`n`个数据点拟合一个圆模型。
- 计算所有数据点到拟合圆的距离,统计距离小于阈值`t`的数据点数量。
- 如果内点数超过`d`,则更新最佳模型和最佳内点数。
- 如果达到最大迭代次数,则停止迭代。
3. **输出最佳模型**:
- 返回具有最多内点的圆模型。
以下是一个简单的Python示例代码,使用RANSAC算法拟合二维圆:
```python
import numpy as np
def fit_circle(points):
# 随机选择3个点来拟合圆
idx = np.random.choice(len(points), 3, replace=False)
x1, y1 = points[idx[0]]
x2, y2 = points[idx[1]]
x3, y3 = points[idx[2]]
# 计算圆心和半径
a = x1*(y2 - y3) - y1*(x2 - x3) + x2*y3 - x3*y2
b = (x1**2 + y1**2)*(y3 - y2) + (x2**2 + y2**2)*(y1 - y3) + (x3**2 + y3**2)*(y2 - y1)
c = (x1**2 + y1**2)*(x2 - x3) + (x2**2 + y2**2)*(x3 - x1) + (x3**2 + y3**2)*(x1 - x2)
x0 = -b/(2*a)
y0 = -c/(2*a)
r = np.sqrt((x1 - x0)**2 + (y1 - y0)**2)
return x0, y0, r
def ransac_circle(points, n_iter, t, d):
best_model = None
best_inliers = []
for _ in range(n_iter):
x0, y0, r = fit_circle(points)
distances = np.abs(np.sqrt((points[:, 0] - x0)**2 + (points[:, 1] - y0)**2) - r)
inliers = points[distances < t]
if len(inliers) > len(best_inliers):
best_model = (x0, y0, r)
best_inliers = inliers
if len(best_inliers) >= d:
break
return best_model
# 示例数据
np.random.seed(0)
points = np.random.uniform(0, 10, (100, 2))
# 添加一些噪声
points = points + np.random.normal(0, 0.5, (100, 2))
# 添加一些异常值
points = np.vstack((points, np.random.uniform(0, 10, (20, 2))))
# RANSAC拟合圆
n_iter = 1000
t = 0.5
d = 80
circle = ransac_circle(points, n_iter, t, d)
print("圆心:", circle[0], circle[1])
print("半径:", circle[2])
```
阅读全文
相关推荐
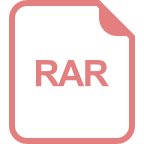
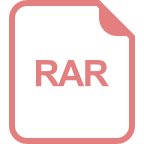
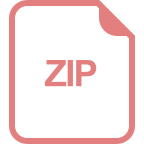





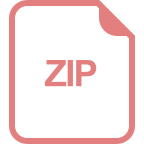
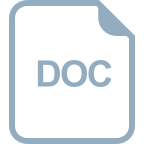





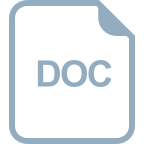



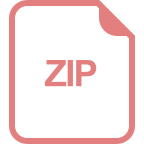