c 语音设计最多能存储 10 个元素的循环队列,并完成初始化、入队、出队、判空、判满、计算元素个数等六个基本操作
时间: 2024-10-10 09:04:45 浏览: 22
在C语言中,我们可以使用数组或者动态内存分配结合指针来实现一个可以存储10个元素的循环队列。这里是一个简单的实现:
```c
typedef struct {
int* queue; // 队列数组
int front; // 队首位置,从0开始
int rear; // 队尾位置,从0开始,取模10是因为循环队列特性
int capacity; // 队列容量
} Queue;
// 初始化函数
void initQueue(Queue* q, int size) {
if (size > 0) {
q->queue = (int*)malloc(size * sizeof(int));
q->front = -1;
q->rear = -1;
q->capacity = size;
} else {
printf("Error: Invalid queue size.\n");
}
}
// 入队操作
void enqueue(Queue* q, int value) {
if (q->rear == (q->front + q->capacity - 1) % q->capacity) { // 判满
printf("Error: Queue is full.\n");
} else {
q->rear = (q->rear + 1) % q->capacity;
q->queue[q->rear] = value;
}
}
// 出队操作
int dequeue(Queue* q) {
if (q-1) { // 判空
printf("Error: Queue is empty.\n");
return -1; // 返回特殊值表示队列为空
} else {
int value = q->queue[q->front];
q->front = (q->front + 1) % q->capacity;
return value;
}
}
// 计算元素个数
int getQueueSize(Queue* q) {
if (q->front != q->rear) {
return (q->rear - q->front + q->capacity) % q->capacity; // 循环队列计算元素个数
} else {
return 0; // 如果队列空,则返回0
}
}
// 判断队列是否为空
int isEmpty(Queue* q) {
return q->front == -1;
}
// 判断队列是否已满
int isFull(Queue* q) {
return q->rear == (q->front + q->capacity - 1) % q->capacity;
}
```
这个实现中,我们使用了两个指针`front`和`rear`来跟踪队头和队尾的位置,并利用队列长度的模运算处理循环队列的特性。记得在不再需要队列时,通过`free(q->queue)`释放内存。
阅读全文
相关推荐
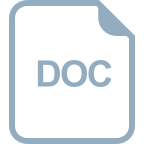
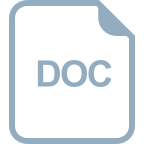
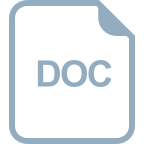














