python编写一个程序,求s=1+(1+2)+(1+2+3)+...+(1+2+3+...+n)。
时间: 2024-04-02 14:30:27 浏览: 113
你可以使用循环来编写一个Python程序来求解这个问题。以下是一个示例程序:
```python
def calculate_sum(n):
total_sum = 0
current_sum = 0
for i in range(1, n+1):
current_sum += i
total_sum += current_sum
return total_sum
n = int(input("请输入一个正整数n:"))
result = calculate_sum(n)
print("结果为:", result)
```
这个程序中,我们定义了一个`calculate_sum`函数,它接受一个正整数`n`作为参数。在函数内部,我们使用两个变量`total_sum`和`current_sum`来分别记录总和和当前的累加和。然后,我们使用一个循环从1到n,依次计算每个累加和,并将其加到总和中。最后,我们返回总和作为结果。
相关问题
编写一个程序,求s=1+2!+3!python
可以使用循环结构和累加器来完成这个任务。具体的代码如下:
```python
n = 3 # 求1+2!+3!,n为3
s = 0 # 初始值为0
for i in range(1, n+1):
factorial = 1 # 阶乘的初始值为1
for j in range(1, i+1):
factorial *= j # 求i的阶乘
s += factorial # 累加每个数的阶乘
print(s) # 输出结果
```
运行结果为9,即1+2!+3!=1+2+6=9。
python编写程序计算sn=1+1/2+1/3....+1/n
以下是两种Python编写程序计算sn=1+1/2+1/3....+1/n的方法:
方法一:使用循环累加
```python
def calc_sn(n):
s = 0
for i in range(1, n+1):
s += 1/i
return s
if __name__ == '__main__':
n = int(input("请输入n的值:"))
sn = calc_sn(n)
print("sn的值为:", sn)
```
方法二:使用递归
```python
def calc_sn(n):
if n == 1:
return 1
else:
return 1/n + calc_sn(n-1)
if __name__ == '__main__':
n = int(input("请输入n的值:"))
sn = calc_sn(n)
print("sn的值为:", sn)
```
阅读全文
相关推荐
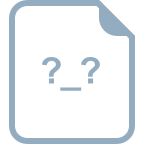
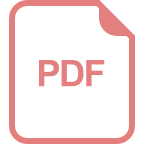
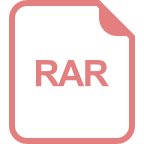













