pcl::PointCloud<pcl::PointXYZ> lidar_cloud; 对其中每个点进行判断
时间: 2024-09-12 13:16:21 浏览: 33
在PCL(Point Cloud Library,点云库)中,`pcl::PointCloud<pcl::PointXYZ>`是一个三维点云数据结构,它表示由一系列 `pcl::PointXYZ` 类型的点组成的数据集合,每个 `pcl::PointXYZ` 点包含三个坐标值 (x, y, z)。当你有这样一个变量 `lidar_cloud`,你可以对其进行操作,比如遍历每个点。
如果你想对每个点进行判断,例如检查某个条件(如是否在某个半径内、颜色是否满足某种阈值等),可以按照以下步骤:
1. 遍历点云:
```cpp
for (const auto& point : lidar_cloud.points) {
const pcl::PointXYZ &p = point;
// 这里可以根据 p.x, p.y, p.z 的值进行判断
if (/* 条件 */) {
// 执行相应的处理
}
}
```
2. 在判断过程中,你可以访问每个点的属性并应用你需要的算法(如计算距离、过滤等):
```cpp
double distance = sqrt(pow(p.x - target_x, 2) + pow(p.y - target_y, 2) + pow(p.z - target_z, 2));
if (distance < radius_threshold) {
// 点在指定范围内
}
```
相关问题
ros2 中将 sensor_msgs::msg::LaserScan ddp; 转换成点云数据 pcl::PointCloud<pcl::PointXYZ> lidar_cloud;
在ROS 2 (Robot Operating System) 中,`sensor_msgs::msg::LaserScan` 类型的数据表示的是激光雷达扫描信息,包含了一系列的测量数据,如角度、强度等。而 `pcl::PointCloud<pcl::PointXYZ>` 是 Point Cloud Library (PCL) 提供的一个结构体,用于存储三维空间中的点云数据,每个点由 x、y 和 z 坐标组成。
要从 `LaserScan` 转换为 `pcl::PointCloud<pcl::PointXYZ>`,你需要对激光雷达的数据进行解析,并将其映射到三维坐标上。以下是一个简化版的步骤:
1. 首先,确保已经包含了所需的库头文件,例如:
```cpp
#include <ros/ros.h>
#include <sensor_msgs/LaserScan.h>
#include <pcl_conversions/pcl_conversions.h>
#include <pcl/point_cloud.h>
#include <pcl/point_types.h>
```
2. 创建一个函数,接收 `sensor_msgs::msg::LaserScan` 数据:
```cpp
void laserScanToPointCloud(const sensor_msgs::msg::LaserScan& scan, pcl::PointCloud<pcl::PointXYZ>& lidar_cloud)
{
// 获取激光扫描范围内的点数
int num_points = scan.ranges.size();
// 初始化点云
lidar_cloud.resize(num_points);
for (size_t i = 0; i < num_points; ++i)
{
double angle = scan.angle_min + i * (scan.angle_increment);
double distance = scan.ranges[i];
// 将角度转换为x,y坐标(假设激光雷达垂直于地面)
float x = distance * cos(angle);
float y = distance * sin(angle);
// 添加z坐标通常为0,因为激光雷达水平扫描
float z = 0;
// 将点添加到点云中
lidar_cloud.points[i].x = x;
lidar_cloud.points[i].y = y;
lidar_cloud.points[i].z = z;
}
}
```
这个函数简单地将激光雷达的线性距离和角度转换为笛卡尔坐标,并放入 `lidar_cloud` 中。
pcl::PointCloud<pcl::PointXYZI>::Ptr &
This is a smart pointer to a point cloud object of type pcl::PointXYZI. It is used to allocate and manage memory for the point cloud object dynamically, and is part of the Point Cloud Library (PCL) in C++.
The point cloud is represented as a set of points in 3D space, where each point has an intensity value in addition to its x, y, and z coordinates. This type of point cloud is often used in lidar and laser scanning applications, where the intensity value represents the reflected laser beam strength at that particular point.
The "Ptr" suffix in the type indicates that this is a smart pointer, which means that it automatically manages the memory allocation and deallocation for the point cloud object. This helps to prevent memory leaks and other memory-related issues that can occur when using raw pointers.
相关推荐
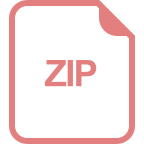
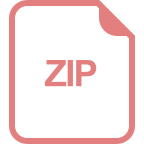
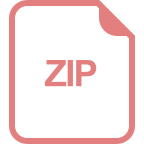












