c++两幅影像配准代码
时间: 2023-12-10 13:33:01 浏览: 37
以下是一份基于OpenCV库的C++图像配准代码:
```c++
#include <opencv2/opencv.hpp>
#include <iostream>
using namespace cv;
using namespace std;
int main(int argc, char** argv)
{
// 读取待配准图像
Mat img1 = imread("img1.jpg");
Mat img2 = imread("img2.jpg");
// 转换为灰度图像
Mat gray1, gray2;
cvtColor(img1, gray1, COLOR_BGR2GRAY);
cvtColor(img2, gray2, COLOR_BGR2GRAY);
// 特征点检测
vector<KeyPoint> keypoints1, keypoints2;
Ptr<FeatureDetector> detector = ORB::create();
detector->detect(gray1, keypoints1);
detector->detect(gray2, keypoints2);
// 特征点描述
Mat descriptors1, descriptors2;
Ptr<DescriptorExtractor> descriptor = ORB::create();
descriptor->compute(gray1, keypoints1, descriptors1);
descriptor->compute(gray2, keypoints2, descriptors2);
// 特征点匹配
vector<DMatch> matches;
Ptr<DescriptorMatcher> matcher = DescriptorMatcher::create("BruteForce-Hamming");
matcher->match(descriptors1, descriptors2, matches);
// 筛选匹配点
double min_dist = 10000, max_dist = 0;
for (int i = 0; i < descriptors1.rows; i++)
{
double dist = matches[i].distance;
if (dist < min_dist) min_dist = dist;
if (dist > max_dist) max_dist = dist;
}
vector<DMatch> good_matches;
for (int i = 0; i < descriptors1.rows; i++)
{
if (matches[i].distance <= max(2 * min_dist, 30.0))
{
good_matches.push_back(matches[i]);
}
}
// 显示匹配结果
Mat img_matches;
drawMatches(img1, keypoints1, img2, keypoints2, good_matches, img_matches);
imshow("matches", img_matches);
waitKey(0);
// 计算变换矩阵
vector<Point2f> points1, points2;
for (int i = 0; i < good_matches.size(); i++)
{
points1.push_back(keypoints1[good_matches[i].queryIdx].pt);
points2.push_back(keypoints2[good_matches[i].trainIdx].pt);
}
Mat H = findHomography(points1, points2, RANSAC);
// 图像配准
Mat img_aligned;
warpPerspective(img1, img_aligned, H, img1.size());
imshow("aligned", img_aligned);
waitKey(0);
return 0;
}
```
该代码使用ORB算法进行特征点检测和描述,使用汉明距离进行特征点匹配,使用RANSAC算法计算变换矩阵,最终使用透视变换将待配准图像进行配准。你可以根据自己的需求进行修改和优化。
相关推荐
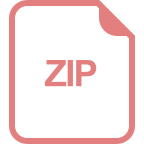
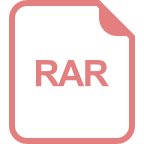
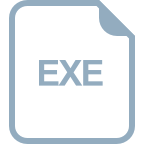














