python 目录下有log文件,从中提取倒数第二行最后一个数据,其中log是以%Y%m%d%H%M%S格式命名,根据数据生成曲线
时间: 2024-12-18 15:19:27 浏览: 1
在Python中,你可以使用`os`, `datetime`, 和 `pandas` 这些库来处理这个问题。首先,你需要遍历目录找到所有以`%Y%m%d%H%M%S`格式的log文件,然后读取每一行,找到倒数第二行并提取最后一个数据。假设数据在每行都是逗号分隔,可以使用正则表达式来解析。
以下是一个简单的步骤:
```python
import os
import re
from datetime import datetime
# 定义函数来提取日期和数据
def extract_data(file_path):
with open(file_path, 'r') as f:
lines = f.readlines()
# 提取倒数第二行
last_second_line = lines[-2]
# 使用正则表达式查找日期和数据
match = re.search(r'(\d{4})(\d{2})(\d{2})\D*(\d{2})(\d{2})(\d{2}).*?(\d+)', last_second_line)
if match:
date_str = f'{match.group(1)}{match.group(2)}{match.group(3)}{match.group(4)}{match.group(5)}{match.group(6)}'
data = int(match.group(7))
return datetime.strptime(date_str, '%Y%m%d%H%M%S'), data
# 遍历目录
dir_path = '/path/to/your/directory' # 替换为你实际的目录路径
files = [f for f in os.listdir(dir_path) if f.endswith('.log') and f.startswith('%Y%m%d%H%M%S')]
# 将数据收集到字典中,键是日期,值是对应的数据
data_points = {}
for file in files:
dt, value = extract_data(os.path.join(dir_path, file))
data_points[dt] = value
# 如果你想生成曲线图,可以使用pandas DataFrame和matplotlib
import pandas as pd
df = pd.DataFrame(list(data_points.items()), columns=['Date', 'Value'])
df['Date'] = pd.to_datetime(df['Date']) # 转换为日期格式
df.set_index('Date', inplace=True)
import matplotlib.pyplot as plt
plt.plot(df['Value'])
plt.title('Log Data Curve')
plt.xlabel('Date')
plt.ylabel('Data Value')
plt.show()
```
阅读全文
相关推荐
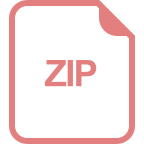
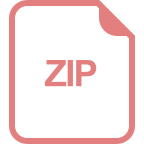
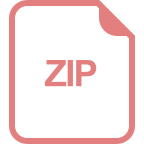











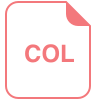




