用C++编写一个程序要求·读并写学生名单 ·任务:编写一个程序,创建一个名为Students的文件。包含学生及其分数的列表。然后读取文件计算并打印平均分。
时间: 2024-12-12 13:24:53 浏览: 4
为了完成这个任务,你可以使用C++的文件流操作、结构体或类来存储学生的姓名和分数,并进行相应的读取和计算。这里是一个简单的示例:
```cpp
#include <iostream>
#include <fstream>
#include <string>
#include <vector>
// 定义一个结构体来表示学生信息
struct Student {
std::string name;
int score;
};
int main() {
// 文件操作
std::ofstream file("Students.txt"); // 创建或打开 Students 文件
if (!file.is_open()) {
std::cerr << "Error opening the file." << std::endl;
return 1;
}
// 假设已有一个学生名字和分数的向量
std::vector<Student> students = {
{"Alice", 90},
{"Bob", 85},
{"Charlie", 95}
};
for (const auto& student : students) {
file << student.name << ": " << student.score << "\n";
}
file.close(); // 关闭文件
// 再次打开文件读取数据并计算平均分
std::ifstream inputFile("Students.txt");
double sum = 0, count = 0;
if (inputFile.is_open()) {
std::string line;
while (std::getline(inputFile, line)) {
size_t pos = line.find(": ");
if (pos != std::string::npos) {
int score = std::stoi(line.substr(pos + 2));
sum += score;
count++;
}
}
inputFile.close();
double average = static_cast<double>(sum) / count;
std::cout << "Average score: " << average << std::endl;
} else {
std::cerr << "Error reading the file." << std::endl;
}
return 0;
}
阅读全文
相关推荐
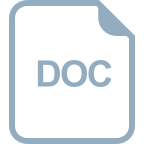
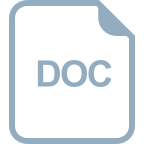
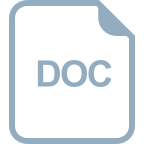
















