python画矩形框
时间: 2024-10-13 11:16:42 浏览: 28
在Python中,通常使用matplotlib、PIL(Pillow)或OpenCV等库来绘制矩形框。这里给出一些基本的例子:
1. **使用matplotlib**:
```python
import matplotlib.pyplot as plt
import numpy as np
def draw_rectangle(x, y, width, height, ax=None):
if ax is None:
fig, ax = plt.subplots()
rect = patches.Rectangle((x, y), width, height, fill=False, edgecolor='red', linewidth=2)
ax.add_patch(rect)
ax.set_xlim([0, width+width])
ax.set_ylim([0, height+height])
return ax
# 示例
ax = draw_rectangle(50, 75, 100, 50)
plt.show()
```
2. **使用PIL(Pillow)**,对于图像操作更为方便:
```python
from PIL import Image, ImageDraw, ImageFont
def draw_rectangle(image, x, y, width, height, color=(255, 0, 0)):
draw = ImageDraw.Draw(image)
draw.rectangle([(x, y), (x + width, y + height)], outline=color)
del draw
return image
# 示例
image = Image.new("RGB", (400, 400), "white")
rect_image = draw_rectangle(image, 50, 50, 100, 100)
rect_image.show()
```
3. **使用OpenCV**:
```python
import cv2
def draw_rectangle_on_image(img, pt1, pt2, color=(0, 0, 255), thickness=2):
cv2.rectangle(img, pt1, pt2, color, thickness)
return img
# 示例
img = np.zeros((400, 400, 3), dtype=np.uint8)
pt1 = (50, 50)
pt2 = (150, 150)
result_img = draw_rectangle_on_image(img, pt1, pt2)
cv2.imshow('Rectangle', result_img)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
以上代码分别展示了如何在图形窗口、图像文件以及OpenCV的BGR图片上绘制矩形框。
阅读全文
相关推荐



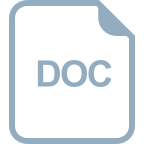







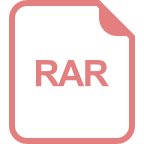
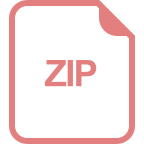
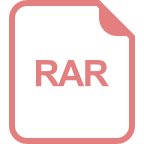
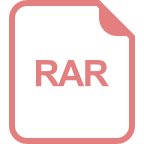