openGL +Python 鼠标拖动画矩形框选出点云数据的感兴趣区域
时间: 2023-06-26 17:07:56 浏览: 76
可以使用 PyOpenGL 库来实现 OpenGL 和 Python 的交互,然后使用 OpenGL 的鼠标事件来实现拖动画矩形框选出点云数据的感兴趣区域。具体实现步骤如下:
1. 导入 PyOpenGL 库和其他必需的库:
```python
from OpenGL.GL import *
from OpenGL.GLUT import *
from OpenGL.GLU import *
from math import *
import numpy as np
```
2. 定义全局变量,包括点云数据、矩形框线条颜色、矩形框坐标、是否拖动矩形框等:
```python
# 点云数据
point_cloud = np.random.rand(100, 3)
# 矩形框线条颜色
rect_color = (1, 0, 0)
# 矩形框坐标
rect_x1, rect_y1, rect_x2, rect_y2 = None, None, None, None
# 是否拖动矩形框
dragging = False
```
3. 定义 OpenGL 的回调函数,包括绘制场景、鼠标事件和键盘事件:
```python
def draw():
glClear(GL_COLOR_BUFFER_BIT | GL_DEPTH_BUFFER_BIT)
glLoadIdentity()
# 绘制点云数据
glColor3f(1, 1, 1)
glPointSize(2)
glBegin(GL_POINTS)
for p in point_cloud:
glVertex3f(p[0], p[1], p[2])
glEnd()
# 绘制矩形框
if rect_x1 is not None and rect_y1 is not None and rect_x2 is not None and rect_y2 is not None:
glColor3f(*rect_color)
glLineWidth(2)
glBegin(GL_LINE_LOOP)
glVertex3f(rect_x1, rect_y1, 0)
glVertex3f(rect_x1, rect_y2, 0)
glVertex3f(rect_x2, rect_y2, 0)
glVertex3f(rect_x2, rect_y1, 0)
glEnd()
glutSwapBuffers()
def mouse(button, state, x, y):
global dragging, rect_x1, rect_y1, rect_x2, rect_y2
if button == GLUT_LEFT_BUTTON and state == GLUT_DOWN:
dragging = True
rect_x1, rect_y1 = x, y
rect_x2, rect_y2 = x, y
elif button == GLUT_LEFT_BUTTON and state == GLUT_UP:
dragging = False
glutPostRedisplay()
def motion(x, y):
global rect_x1, rect_y1, rect_x2, rect_y2
if dragging:
rect_x2, rect_y2 = x, y
glutPostRedisplay()
def keyboard(key, x, y):
if key == b'\x1b':
sys.exit()
```
4. 初始化 OpenGL 窗口,并启动主循环:
```python
glutInit()
glutInitDisplayMode(GLUT_RGBA | GLUT_DOUBLE | GLUT_DEPTH)
glutInitWindowSize(800, 600)
glutCreateWindow("Point Cloud ROI Selection")
glutDisplayFunc(draw)
glutMouseFunc(mouse)
glutMotionFunc(motion)
glutKeyboardFunc(keyboard)
glClearColor(0, 0, 0, 0)
glutMainLoop()
```
运行程序后,可以使用鼠标拖动矩形框来选择点云数据的感兴趣区域。选择完成后,可以通过计算矩形框内的点云数据来提取感兴趣区域。
相关推荐
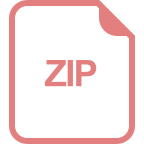













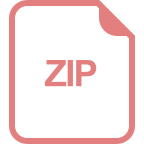
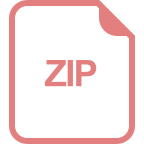