python tensorflow densenet121
时间: 2024-01-09 17:01:38 浏览: 210
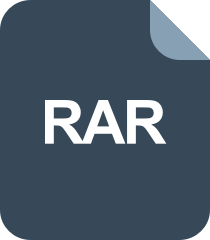
使用 TensorFlow 创建 DenseNet 121
Python是一种高级的编程语言,广泛应用于机器学习和人工智能领域。它具有简洁的语法和强大的库支持,使得开发者能够更加高效地实现复杂的算法和模型。
TensorFlow是一个流行的开源深度学习框架,支持构建和训练各种深度神经网络模型。它为开发者提供了一系列的高层次API,比如Keras,使得构建和训练模型变得更加简单和灵活。
DenseNet是一种深度神经网络模型,最早由Kaiming He等人在2016年提出。与传统的卷积神经网络不同,DenseNet的每个层都与其他层密集连接。这种密集连接能够促进信息流动,使得模型更易训练和学习。DenseNet的一个重要特点是它在较少的参数下能够达到较高的性能,并且对于梯度消失问题有很好的解决办法。
在Python中使用TensorFlow和DenseNet-121模型可以进行各种图像分类和目标识别任务。首先,我们可以使用TensorFlow的API构建一个DenseNet-121模型,然后使用该模型加载和预处理图像数据。接下来,我们可以利用该模型对图像进行分类,得出每个类别的预测概率。最后,我们可以根据预测结果对图像进行分类或者目标识别。
为了实现这些任务,我们还可以借助Python和TensorFlow中提供的其他库和工具,如NumPy和PIL。通过这些工具的配合,我们可以更加高效地完成图像处理和模型训练的相关工作。
阅读全文
相关推荐
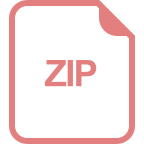
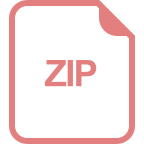
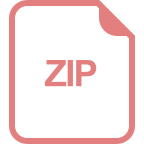
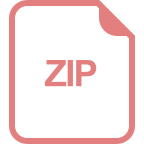
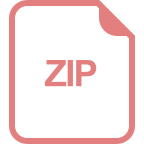
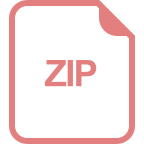
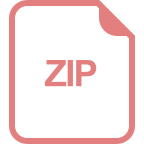
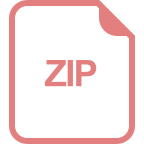
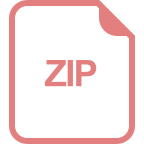
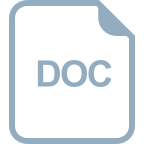
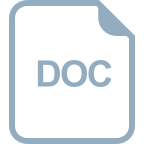





