std::remove(文件名)
时间: 2024-05-21 09:09:10 浏览: 13
std::remove() 是一个 C++ 标准库函数,用于删除文件。当你调用这个函数时,它会尝试删除指定的文件。如果删除成功,它将返回 0;否则,它将返回一个非零值。需要注意的是,该函数只能删除普通文件和空目录,而不能删除非空目录。
例如,以下代码可以删除名为 filename 的文件:
```
#include <cstdio>
int main() {
const char* filename = "example.txt";
int result = std::remove(filename);
if (result == 0) {
printf("File deleted successfully.\n");
} else {
printf("Failed to delete file.\n");
}
return 0;
}
```
相关问题
使用std::string content((std::istreambuf_iterator<char>(ifs)), (std::istreambuf_iterator<char>())); 并且不输出最后一行
您可以使用 `std::string` 的 `substr` 函数来删除最后一行。这个函数接受两个参数,第一个参数是起始位置,第二个参数是要删除的字符数。
以下是使用 `std::istreambuf_iterator` 和 `std::string` 的 `substr` 函数来获取文件内容并删除最后一行的示例代码:
```c++
#include <iostream>
#include <fstream>
#include <string>
using namespace std;
int main() {
string filename = "example.txt";
string content = "";
ifstream input(filename);
if (input) {
content.assign((istreambuf_iterator<char>(input)), (istreambuf_iterator<char>()));
}
input.close();
// Find the last newline character
size_t last_newline_index = content.find_last_of("\n");
// Remove the last line from the content string
if (last_newline_index != string::npos) {
content = content.substr(0, last_newline_index);
}
cout << "Content without the last line:\n" << content << endl;
return 0;
}
```
在这个示例中,我们首先定义了文件名和一个字符串来存储文件内容。我们打开文件并检查文件是否成功打开。如果文件已成功打开,则使用 `std::istreambuf_iterator` 一次性获取整个文件的内容,并将其存储在内容字符串中。
接下来,我们使用 `find_last_of` 函数找到最后一个换行符的位置。如果找到了该字符,则使用 `substr` 函数删除最后一行。最后,我们关闭文件并输出不包括最后一行的内容字符串。
请注意,在使用 `substr` 函数时,我们将位置 0 作为第一个参数,以确保字符串中的所有内容都被包含在内。
inline bool LoadConfigFile(const std::string& filename, std::string& content, const unsigned char* aes_key) { // 解密文件 DecryptFile(filename, "config.txt", aes_key); // 打开文件 std::ifstream file("config.txt"); if (!file) { std::cerr << "Failed to open config file: " << filename << std::endl; return false; } // 读取文件内容 std::getline(file, content); // 关闭文件 file.close(); // 删除解密后的文件 // remove("config.txt"); return true; } if (!LoadConfigFile(CONFIG_FILE, content, aes_key)) { // 如果加载失败,说明配置文件不存在或已被篡改,需要重新创建 content = GetCurrentTimestampString(); SaveConfigFile(CONFIG_FILE, content, aes_key); } Failed to open input file: config.txt.enc
这段代码中调用了 `DecryptFile` 函数,这个函数的作用是将加密的文件解密,解密后的文件名为 "config.txt"。但是,如果解密失败或者解密后的文件不存在,则会导致 `std::ifstream` 打开文件失败,从而产生 "Failed to open config file" 的错误信息。
因此,你需要检查以下几点:
1. 确认加密的文件名是否为 "config.txt.enc"。
2. 确认解密后的文件名是否为 "config.txt"。
3. 确认解密后的文件是否存在,可以通过检查文件是否存在的方式来验证。
如果以上的检查都没有问题,那么你需要检查文件的读取权限是否正确。
相关推荐
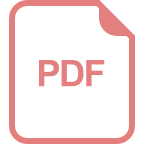
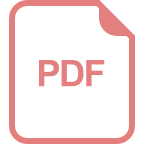
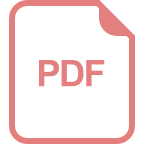
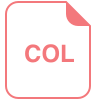
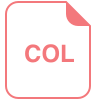










