写一个C++高精度最小公倍数的代码
时间: 2024-11-05 22:31:43 浏览: 8
在C++中,实现高精度计算的最小公倍数(LCM,Least Common Multiple)通常需要借助于两个数的最大公约数(GCD,Greatest Common Divisor),因为根据数学原理,两个数的乘积等于它们的最大公约数和最小公倍数的乘积。下面是一个简单的示例,使用辗转相除法(欧几里得算法)来求最大公约数,并通过递归来求最小公倍数:
```cpp
#include <iostream>
#include <vector>
// 定义一个大整数类,这里假设使用vector存储每一位
class BigInt {
public:
BigInt() : value({0}) {}
BigInt(int num) { *this = num; }
BigInt operator=(int num) {
std::vector<int> digits;
while (num > 0) {
digits.push_back(num % 10);
num /= 10;
}
reverse(digits.begin(), digits.end());
value = digits;
}
// 辗转相除法求最大公约数
BigInt gcd(const BigInt& other) const {
if (other == 0)
return *this;
else
return other.gcd(*this % other);
}
private:
std::vector<int> value;
};
// 根据最大公约数计算最小公倍数
BigInt lcm(const BigInt& a, const BigInt& b) {
return a * b / a.gcd(b);
}
int main() {
BigInt num1(12345), num2(67890);
BigInt result = lcm(num1, num2);
std::cout << "The least common multiple of " << num1 << " and " << num2 << " is: " << result << std::endl;
return 0;
}
```
在这个例子中,我们首先定义了一个`BigInt`类用于表示大整数,然后实现了`gcd`函数来求两个数的最大公约数,最后利用这个函数在`lcm`函数中计算最小公倍数。
阅读全文
相关推荐
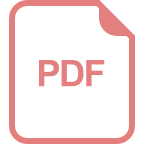
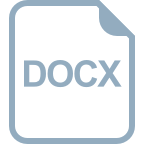
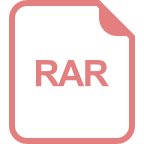

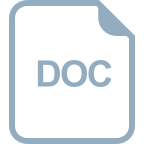
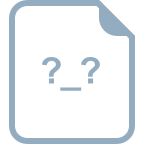
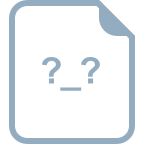
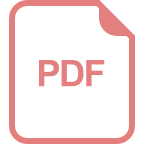
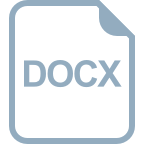
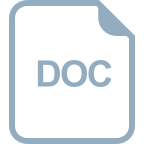
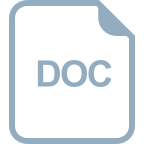
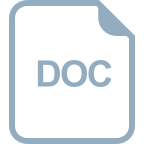
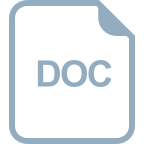
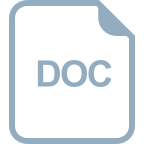
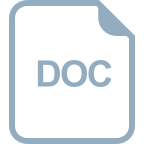
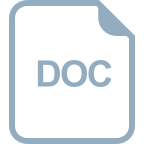
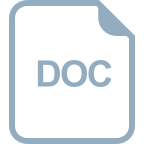
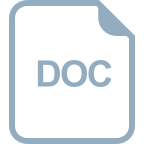
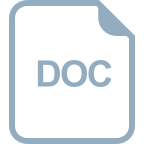