numpy.uint16转tensor
时间: 2023-10-19 12:47:13 浏览: 298
要将numpy数组的数据类型从`numpy.uint16`转换为PyTorch的Tensor,可以使用`torch.from_numpy()`函数。以下是一个示例:
```python
import numpy as np
import torch
# 创建一个numpy数组
arr = np.array([1, 2, 3], dtype=np.uint16)
# 将numpy数组转换为torch tensor
tensor = torch.from_numpy(arr)
```
在上面的示例中,我们首先创建了一个名为`arr`的numpy数组,数据类型为`numpy.uint16`。然后,我们使用`torch.from_numpy()`函数将该数组转换为PyTorch的Tensor,存储在变量`tensor`中。
请注意,转换后的Tensor将具有与原始numpy数组相同的数据类型。
相关问题
D:\python3.10\lib\site-packages\torch\nn\modules\linear.py:96: UserWarning: Failed to initialize NumPy: module compiled against API version 0x10 but this version of numpy is 0xf . Check the section C-API incompatibility at the Troubleshooting ImportError section at https://numpy.org/devdocs/user/troubleshooting-importerror.html#c-api-incompatibility for indications on how to solve this problem . (Triggered internally at ..\torch\csrc\utils\tensor_numpy.cpp:84.) self.weight = Parameter(torch.empty((out_features, in_features), **factory_kwargs)) Traceback (most recent call last): File "C:\Users\admin\PycharmProjects\dl1test\main.py", line 46, in <module> data_train = torch.tensor(x_train).float() RuntimeError: Could not infer dtype of numpy.uint8
这个警告提示是因为 NumPy 的版本和 Torch 包中的线性层模块不兼容,需要通过查看在 https://numpy.org/devdocs/user/troubleshooting-importerror.html 中关于 C-API 不兼容的部分来解决 ImportError 的问题。
tensor=(tensor[i][j]* 255.).cpu().numpy().clip(0, 255).astype(np.uint8) AttributeError: 'numpy.float64' object has no attribute 'cpu' 出现这个问题应该怎么处理
这个错误是因为你尝试在一个numpy.float64对象上调用`cpu()`方法,而`cpu()`方法是PyTorch张量对象的方法,不适用于numpy数组。
要解决这个问题,你可以使用`torch.from_numpy()`方法将numpy数组转换为PyTorch张量,然后再进行操作。下面是修改后的代码:
```python
import numpy as np
import torch
from PIL import Image
# 假设tensor大小为[2, 1280, 64, 64]
tensor = torch.randn(2, 1280, 64, 64)
for i in range(tensor.size(0)):
for j in range(tensor.size(1)):
# 将tensor转换为图片
img_tensor = (torch.from_numpy(tensor[i][j]) * 255.).numpy().clip(0, 255).astype(np.uint8)
new_image = Image.fromarray(img_tensor)
# 保存图片到本地,命名方式为 "image_i_j.png"
new_image.save(f"image_{i}_{j}.png")
```
通过使用`torch.from_numpy()`将numpy数组转换为PyTorch张量,然后再进行操作,就可以避免这个错误。
阅读全文
相关推荐
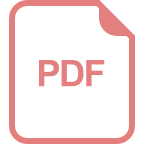
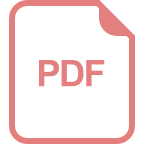














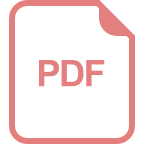