多项式 a 和 b 相加,建立单链表多项式.a+b,和结果保存在 a, 销毁b全部代码
时间: 2024-12-23 12:24:54 浏览: 9
在C#中,我们可以使用类来表示多项式及其节点,然后创建一个链表结构来存储它们。这里我会为你提供一个简单的实现,假设每个多项式的节点包含系数和指数:
```csharp
using System;
using System.Collections.Generic;
// 定义一个多项式的节点
public class PolynomialTerm
{
public int Coefficient { get; set; }
public int Exponent { get; set; }
// 构造函数
public PolynomialTerm(int coefficient, int exponent)
{
Coefficient = coefficient;
Exponent = exponent;
}
}
// 定义多项式类,包含一个双向链表用于存储项
public class Polynomial
{
private LinkedList<PolynomialTerm> terms;
private bool isDestroyed;
// 初始化构造函数
public Polynomial()
{
terms = new LinkedList<PolynomialTerm>();
}
// 添加项到链表
public void AddTerm(PolynomialTerm term)
{
if (term != null)
terms.AddLast(term);
}
// 将两个多项式相加并把结果存入a
public void Add(Polynomial b)
{
if (b == null || isDestroyed) return;
foreach (var term in b.terms)
{
var sumTerm = new PolynomialTerm(0, term.Exponent);
if (terms.Count > 0 && terms.Last.Value.Exponent == term.Exponent)
sumTerm.Coefficient += terms.Last.Value.Coefficient + term.Coefficient;
else
sumTerm.Coefficient = term.Coefficient;
terms.AddLast(sumTerm);
}
}
// 销毁链表(这里只是设置标记,实际要确保链表被清理)
public void Destroy()
{
isDestroyed = true;
}
}
// 使用示例
class Program
{
static void Main(string[] args)
{
Polynomial a = new Polynomial();
a.AddTerm(new PolynomialTerm(2, 0)); // a = 2x^0
a.AddTerm(new PolynomialTerm(3, 1)); // a = 2x^0 + 3x^1
Polynomial b = new Polynomial();
b.AddTerm(new PolynomialTerm(4, 0)); // b = 4x^0
b.AddTerm(new PolynomialTerm(5, 1)); // b = 4x^0 + 5x^1
a.Add(b); // a = (2+4)x^0 + (3+5)x^1 = 6x^0 + 8x^1
Console.WriteLine("a after addition: " + a.ToString());
// 销毁b
b.Destroy();
// 注意:这只是演示销毁标记,链表可能需要进一步处理
Console.WriteLine($"Is b destroyed? {b.isDestroyed}");
}
// 示例:字符串形式表示多项式
public static string ToString(this Polynomial polynomial)
{
string result = "";
foreach (var term in polynomial.terms)
{
result += $"{term.Coefficient}x^{" + term.Exponent + "}";
if (term.Next != null) result += " + ";
}
return result.TrimEnd(' ', '+');
}
}
```
在这个例子中,我们没有真正地销毁`b`链表,而是通过`isDestroyed`字段标记了`b`是否已经被销毁。如果需要完全删除链表,你需要遍历并移除链表中的所有元素。
阅读全文
相关推荐
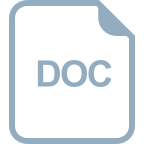
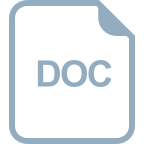
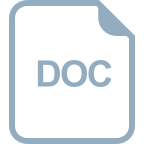


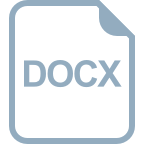
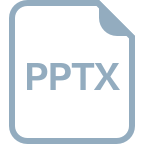
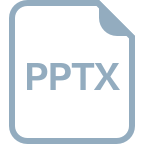
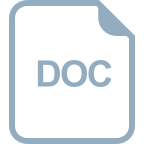
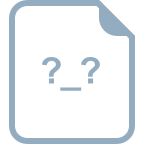
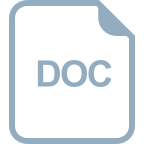
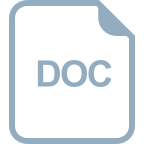
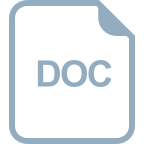





