编写程序,完成手机计算器中表达式的求解(难度分为四级)基本要求:无括号、整数用c写
时间: 2024-10-12 21:07:34 浏览: 89
在C语言中,编写一个基础的手机计算器功能,用于解决无括号的整数加减乘除表达式,可以采用递归解析法,也就是栈的应用。这里我们简化处理只考虑四则运算,忽略优先级规则。下面是一个基础版本的示例:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
typedef struct Node {
int value;
char op;
struct Node* left;
struct Node* right;
} Node;
Node* createNumber(int val) {
Node* node = (Node*)malloc(sizeof(Node));
node->value = val;
node->left = NULL;
node->right = NULL;
node->op = '\0';
return node;
}
Node* createOperator(char op, Node* left, Node* right) {
Node* node = (Node*)malloc(sizeof(Node));
node->value = 0;
node->op = op;
node->left = left;
node->right = right;
return node;
}
int applyOp(int a, int b, char op) {
switch (op) {
case '+': return a + b;
case '-': return a - b;
case '*': return a * b;
case '/': return a / b; // 检查除数是否为0
default: return 0; // 错误操作符
}
}
int evaluateExpression(char* expression) {
Node* root = createNumber(strtol(expression, NULL, 10));
char* token = strtok(expression, " ");
while(token != NULL) {
if(isdigit(*token)) {
Node* right = createNumber(strtol(token, NULL, 10));
Node* current = root;
while(current->right != NULL) {
current = current->right;
}
current->right = right;
} else {
Node* right = root->right;
Node* left = root->left;
root->right = createOperator(*token, left, right);
root = root->right;
}
token = strtok(NULL, " ");
}
return applyOp(root->value, root->right->value, root->op);
}
int main() {
char input[50];
printf("Enter an arithmetic expression without parentheses (e.g., 4 5 +): ");
fgets(input, sizeof(input), stdin);
input[strlen(input) - 1] = '\0'; // remove newline character
int result = evaluateExpression(input);
printf("Result: %d\n", result);
return 0;
}
阅读全文
相关推荐
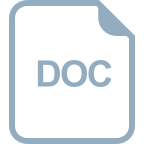
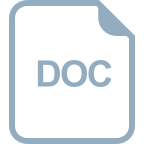
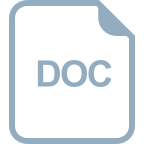

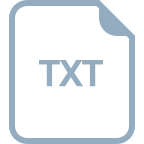








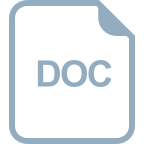
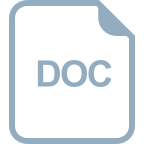
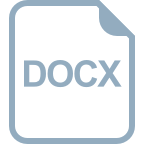
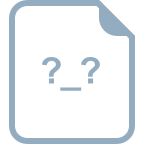