python 八数码 深度优先搜索
时间: 2023-11-20 14:57:51 浏览: 128
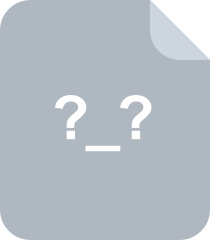
八数码深度优先搜索算法

以下是使用Python实现深度优先搜索算法解决八数码问题的示例代码:
```python
# 定义八数码问题的初始状态和目标状态
start_state = [2, 8, 3, 1, 6, 4, 7, 0, 5]
goal_state = [1, 2, 3, 8, 0, 4, 7, 6, 5]
# 定义节点类
class Node:
def __init__(self, state, parent, action):
self.state = state
self.parent = parent
self.action = action
def expand(self):
# 定义移动操作
def move_up(state):
new_state = state[:]
index = new_state.index(0)
if index not in [0, 1, 2]:
new_state[index - 3], new_state[index] = new_state[index], new_state[index - 3]
return new_state
else:
return None
def move_down(state):
new_state = state[:]
index = new_state.index(0)
if index not in [6, 7, 8]:
new_state[index + 3], new_state[index] = new_state[index], new_state[index + 3]
return new_state
else:
return None
def move_left(state):
new_state = state[:]
index = new_state.index(0)
if index not in [0, 3, 6]:
new_state[index - 1], new_state[index] = new_state[index], new_state[index - 1]
return new_state
else:
return None
def move_right(state):
new_state = state[:]
index = new_state.index(0)
if index not in [2, 5, 8]:
new_state[index + 1], new_state[index] = new_state[index], new_state[index + 1]
return new_state
else:
return None
# 调用移动操作,生成新节点
successors = []
for move in [move_up, move_down, move_left, move_right]:
new_state = move(self.state)
if new_state is not None:
successors.append(Node(new_state, self, move))
return successors
# 定义深度优先搜索算法
def dfs(start_state, goal_state):
start_node = Node(start_state, None, None)
if start_state == goal_state:
return start_node
frontier = [start_node]
explored = set()
while frontier:
node = frontier.pop()
explored.add(tuple(node.state))
for child in node.expand():
if tuple(child.state) not in explored:
if child.state == goal_state:
return child
frontier.append(child)
return None
# 调用深度优先搜索算法,并输出结果
result_node = dfs(start_state, goal_state)
if result_node is None:
print("No solution found.")
else:
path = []
node = result_node
while node is not None:
path.append(node)
node = node.parent
path.reverse()
print("Solution found with", len(path) - 1, "moves:")
for i, node in enumerate(path):
if i == 0:
print("Start state:")
elif i == len(path) - 1:
print("Goal state:")
else:
print("Move", i, ":", node.action.__name__)
print(node.state[0:3])
print(node.state[3:6])
print(node.state[6:9])
print("")
```
运行以上代码,即可使用深度优先搜索算法解决八数码问题。
阅读全文
相关推荐
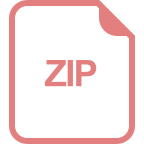
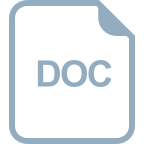









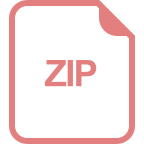
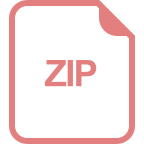


